How to show Image from Base64 in Flutter
Base64 is an encoding scheme that can carry data stored in binary formats. The application of base64 string is common in web and mobile app development. In this blog post, let’s learn how to display an image from base64 in Flutter.
The Image.memory constructor helps to display images from bytes. Hence, we have to convert the base64 string to bytes using dart:convert.
See the code snippet given below.
import 'dart:convert';
import 'dart:typed_data';
String b64 =
'iVBORw0KGgoAAAANSUhEUgAAADMAAAAzCAYAAAA6oTAqAAAAEXRFWHRTb2Z0d2FyZQBwbmdjcnVzaEB1SfMAAABQSURBVGje7dSxCQBACARB+2/ab8BEeQNhFi6WSYzYLYudDQYGBgYGBgYGBgYGBgYGBgZmcvDqYGBgmhivGQYGBgYGBgYGBgYGBgYGBgbmQw+P/eMrC5UTVAAAAABJRU5ErkJggg==';
Uint8List bytesImage = const Base64Decoder().convert(b64);
Image.memory(bytesImage, width: 200, height: 200)
Following is the complete code for Flutter base64 Image example.
import 'dart:convert';
import 'dart:typed_data';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
String b64 =
'iVBORw0KGgoAAAANSUhEUgAAADMAAAAzCAYAAAA6oTAqAAAAEXRFWHRTb2Z0d2FyZQBwbmdjcnVzaEB1SfMAAABQSURBVGje7dSxCQBACARB+2/ab8BEeQNhFi6WSYzYLYudDQYGBgYGBgYGBgYGBgYGBgZmcvDqYGBgmhivGQYGBgYGBgYGBgYGBgYGBgbmQw+P/eMrC5UTVAAAAABJRU5ErkJggg==';
Uint8List bytesImage = const Base64Decoder().convert(b64);
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Base64 Image Example'),
),
body: Center(child: Image.memory(bytesImage, width: 200, height: 200)));
}
}
And you will get the following output.
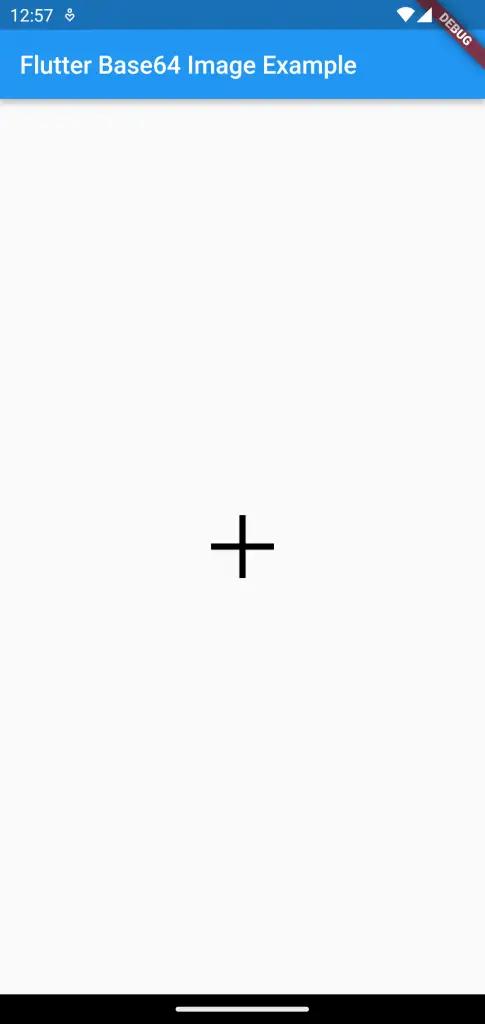
That’s how you show image from base64 data in Flutter.