How to set Width and Height to ElevatedButton in Flutter
EleavetedButton is one of the most used widgets in Flutter. It helps to add a pretty button with the Material design. In this short Flutter tutorial, let’s learn how to set fixed width and height for ElevatedButton.
By default, the size of the ElevatedButton is determined by its content such as Text, Icon etc. The factors such as padding also affect the size. We can give a fixed size to ElevatedButton using the fixedSize property of ElevatedButton.styleFrom method.
See the following code snippet.
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(fixedSize: const Size(150, 70)),
child: const Text(' Elevated Button'))
We give the ElevatedButton a width of 150 and a height of 70 using the Size class. Following is the output.
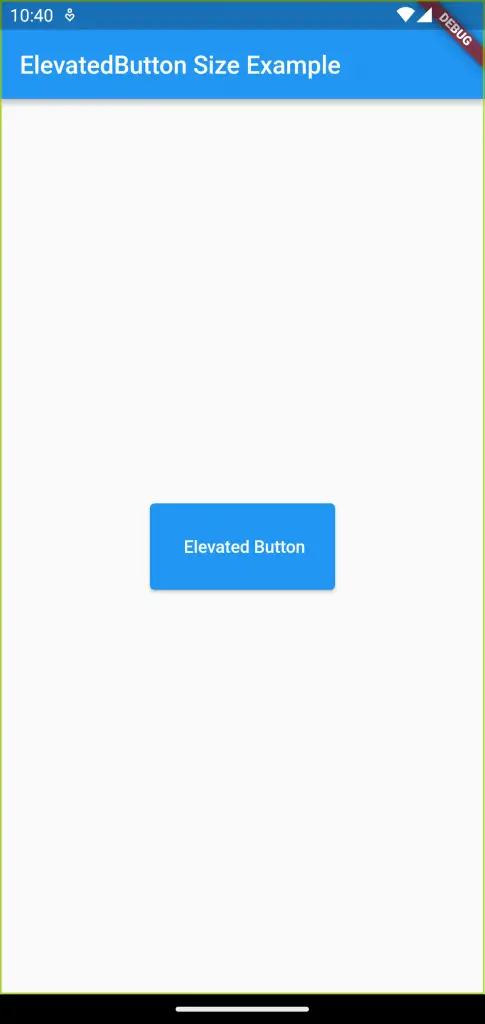
Following is the full code for this ElevatedButton width and height example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ElevatedButton Size Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(fixedSize: const Size(150, 70)),
child: const Text(' Elevated Button'))));
}
}
That’s how you set fixed size for ElevatedButton in Flutter.