How to set TextField Width and Height in Flutter
The TextField widget in Flutter helps to create an input where users can enter data using the keyboard. In this blog post, let’s learn how to set the width and height for TextField in Flutter.
There are no direct properties for TextField to change the default height and width of the TextField. But you can use widgets such as SizedBox, Container, etc as parents to define size and width.
See the code snippet given below.
SizedBox(
width: 200,
height: 40,
child: TextField(
decoration: const InputDecoration(border: OutlineInputBorder()),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
)
You will get the following output.
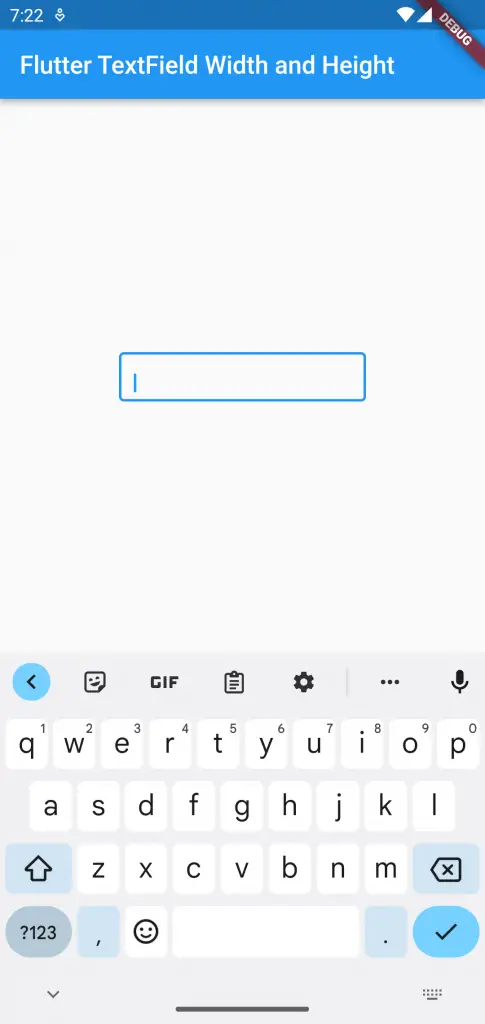
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Width and Height'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: SizedBox(
width: 200,
height: 40,
child: TextField(
decoration: const InputDecoration(border: OutlineInputBorder()),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
)));
}
}
That’s how you change TextField height and width in Flutter.