How to set Padding for Elevated Button in Flutter
Padding is an important aspect that determines the clarity of your mobile app UI. In this Flutter tutorial, let’s check how to apply padding to the ElevatedButton widget.
You can set padding to the elevated button using the Padding class. Make Padding widget parent to the elevated button.
Padding(
padding: EdgeInsets.all(16.0),
child: ElevatedButton(
child: Text('Elevated Button Padding'),
onPressed: () {
print('Pressed');
},
))
Following is the complete example.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Elevated Button Padding Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
const FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Elevated Button with Padding')),
body: Container(
child: Padding(
padding: EdgeInsets.all(20.0),
child: ElevatedButton(
child: Text('Elevated Button Padding'),
onPressed: () {
print('Pressed');
},
style: ElevatedButton.styleFrom(
shape: new RoundedRectangleBorder(
borderRadius: new BorderRadius.circular(20.0),
),
),
))));
}
}
See the output given below. You can see the padding around the button.
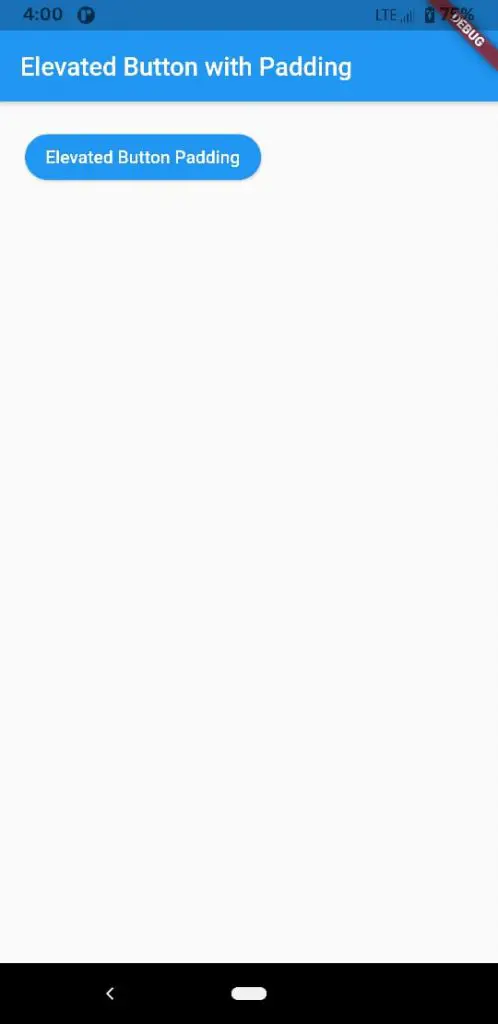
That’s how you add Padding to ElevatedButton.
Sometimes, you may want to change the inner padding of the elevated button. In such cases, make the Padding widget the child of ElevatedButton. See the code snippet given below.
ElevatedButton(
style: ElevatedButton.styleFrom(elevation: 10),
onPressed: () {},
child: const Padding(
padding: EdgeInsets.all(20.0),
child: Text('Elevated Button Padding')),
)
Adding inner padding will result in increasing the size of the button. The output is given below.
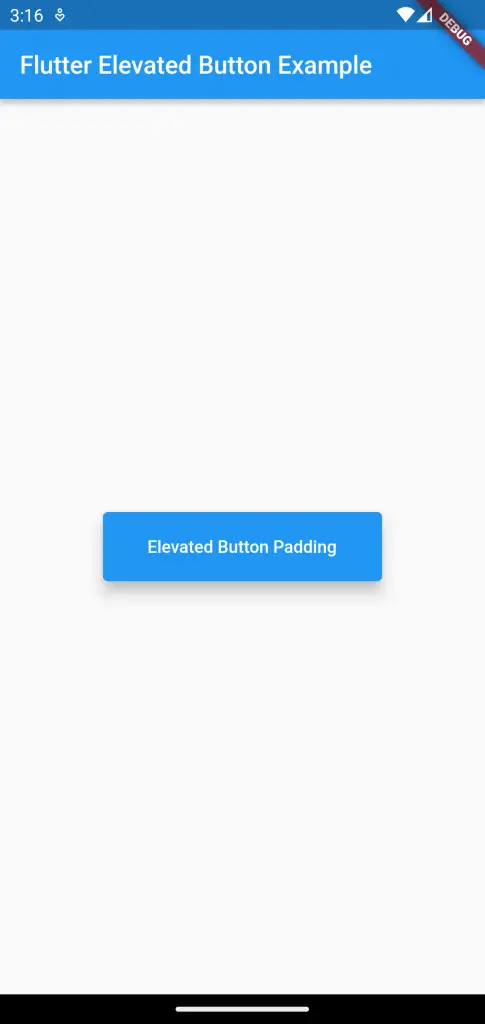
Following is the complete code for ElevatedButton inner padding example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Elevated Button Example',
),
),
body: Center(
child: ElevatedButton(
style: ElevatedButton.styleFrom(elevation: 10),
onPressed: () {},
child: const Padding(
padding: EdgeInsets.all(20.0),
child: Text('Elevated Button Padding')),
)));
}
}
I hope this Flutter ElevatedButton padding tutorial is helpful for you.