How to set Linear Progress Indicator Height in Flutter
Progress indicators are important UI components of a mobile app. In this Flutter tutorial, let’s learn how to set linear progress indicator height.
The LinearProgressIndicator widget helps you to create a horizontal progress indicator in Flutter. It has a property named minHeight that helps to set height.
See the following code snippet.
LinearProgressIndicator(
backgroundColor: Colors.cyan,
color: Colors.red,
minHeight: 10,
)
You will get the following output.
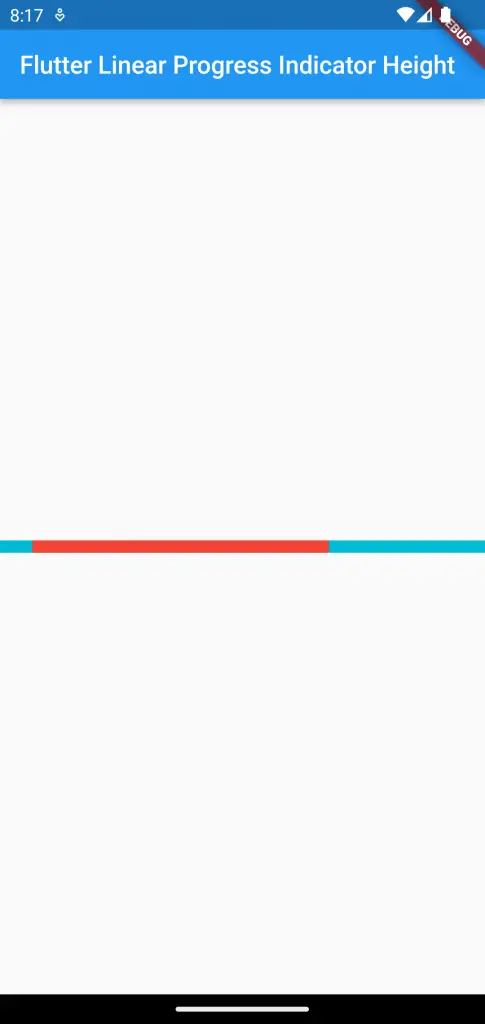
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Linear Progress Indicator Height'),
),
body: const Center(
child: LinearProgressIndicator(
backgroundColor: Colors.cyan,
color: Colors.red,
minHeight: 10,
)));
}
}
That’s how you change the height of LinearProgressIndicator in Flutter.
If you are looking for the circular progress indicator size then see this Flutter tutorial.