How to set Gradient Background for Floating Action Button in Flutter
Gradients have the ability to make UI components fancier. In this blog post, let’s check how to add a Floating Action Button with a gradient background in Flutter.
The FloatingActionButton widget doesn’t have a direct property to create a gradient background. So, we should look for adding another widget that supports a gradient background.
The Container widget can make use of BoxDecoration class and create the gradient. See the code snippet given below.
Container(
decoration: const BoxDecoration(
boxShadow: [
BoxShadow(
color: Colors.grey,
offset: Offset(0, 2.0), //(x,y)
blurRadius: 6.0,
),
],
gradient: LinearGradient(
colors: [
Colors.cyan,
Colors.green,
],
),
shape: BoxShape.circle,
),
child: FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.transparent,
elevation: 0,
child: const Icon(Icons.add)),
),
As you see, the elevation of FAB is set as zero, and the background color is transparent. The BoxShadow class is used to create shadows. We created a linear gradient of cyan and green colors.
Following is the output.
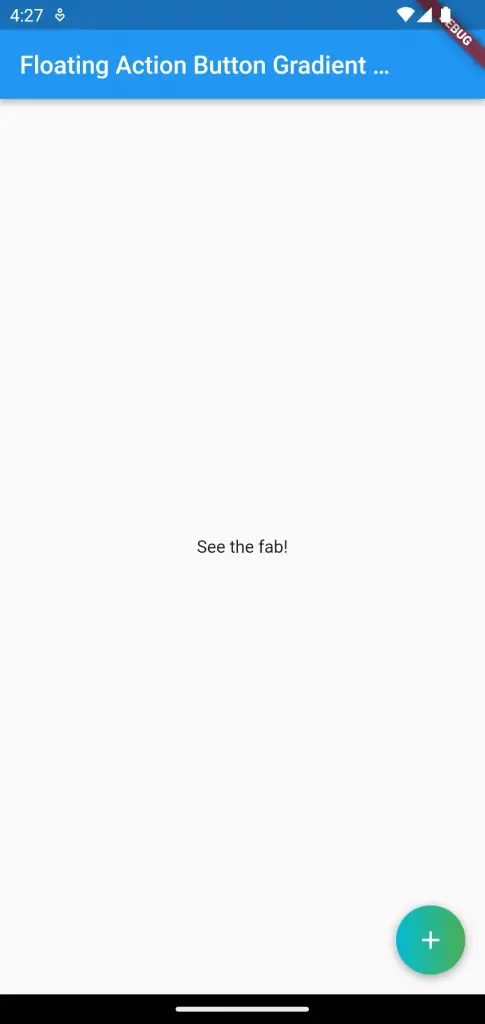
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button Gradient Background'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: Container(
decoration: const BoxDecoration(
boxShadow: [
BoxShadow(
color: Colors.grey,
offset: Offset(0, 2.0), //(x,y)
blurRadius: 6.0,
),
],
gradient: LinearGradient(
colors: [
Colors.cyan,
Colors.green,
],
),
shape: BoxShape.circle,
),
child: FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.transparent,
elevation: 0,
child: const Icon(Icons.add)),
),
);
}
}
That’s how you set the Floating Action Button gradient background in Flutter.