How to set Gradient Background for Card in Flutter
The Card widget is a useful widget to display information neatly in Flutter. In this Flutter tutorial, let’s learn how to set a gradient background for a Card.
There’s no direct way to set the gradient background in the Card widget. So, we should make use of the Container widget and the BoxDecoration class.
You may also check out: How to set image background for Card in Flutter.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(10),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
clipBehavior: Clip.antiAliasWithSaveLayer,
elevation: 5,
child: Container(
decoration: const BoxDecoration(
gradient:
LinearGradient(colors: [Colors.greenAccent, Colors.cyanAccent]),
),
child: const ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
),
),
The LinearGradient is used to show the gradient background with multiple colors.
You will get the following output.
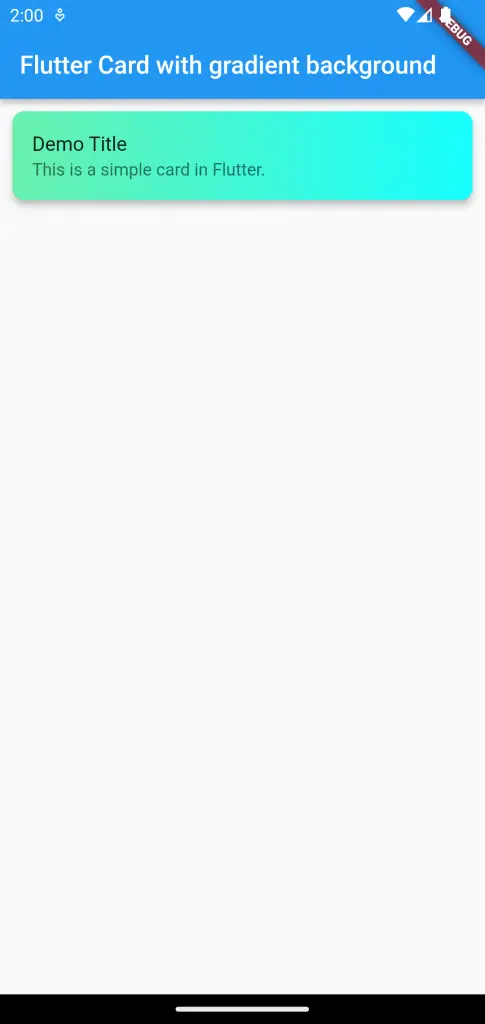
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card with gradient background'),
),
body: Card(
margin: const EdgeInsets.all(10),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(10)),
clipBehavior: Clip.antiAliasWithSaveLayer,
elevation: 5,
child: Container(
decoration: const BoxDecoration(
gradient:
LinearGradient(colors: [Colors.greenAccent, Colors.cyanAccent]),
),
child: const ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
),
),
);
}
}
That’s how you add a gradient background to the Card in Flutter.