How to set Card Elevation in Flutter
The Card widget is one of the commonly used widgets in Flutter. In this blog post, let’s check how to change the elevation to the Card easily in Flutter.
The elevation is the distance from the surface and it decides the size of the shadows too. Hence, increasing elevation causes an increase in the shadows.
The default elevation of the Card is 1.0. You can change the elevation using the elevation property.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(20),
elevation: 10,
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
You will get the following output.
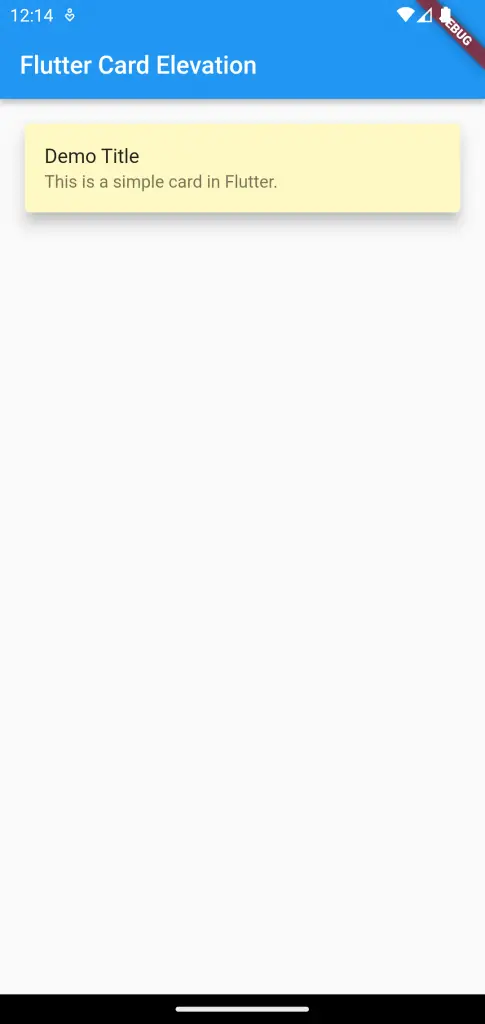
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card Elevation'),
),
body: Card(
margin: const EdgeInsets.all(20),
elevation: 10,
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
);
}
}
You may also check out How to change Card shadow color
That’s how you change Card elevation in Flutter.