How to Reload WebView in Flutter
WebView allows you to display web content within your mobile app. Sometimes, there may be situations where the WebView needs to be reloaded, such as when the web content changes or when the user wants to refresh the page.
In this blog post, we will learn how to reload WebView in Flutter. We use the webview_flutter package in this tutorial. You can install the package using the following command.
flutter pub add webview_flutter
You can import WebView as given below.
import 'package:webview_flutter/webview_flutter.dart';
The package comes with two parts: WebViewController and WebViewWidget. The WebViewController class has the reload method that helps to refresh WebView with the current URL.
IconButton(
icon: const Icon(Icons.refresh),
onPressed: () async {
controller.reload();
},
)
Following is the complete Flutter WebView reload example where the current URL is reloaded when the icon button given in the AppBar is pressed.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
var loadingPercentage = 0;
@override
void initState() {
super.initState();
controller = WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
});
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView example'),
actions: [
IconButton(
icon: const Icon(Icons.refresh),
onPressed: () async {
controller.reload();
},
),
],
),
body: Stack(
children: [
WebViewWidget(
controller: controller,
),
if (loadingPercentage < 100)
LinearProgressIndicator(
value: loadingPercentage / 100.0,
),
],
));
}
}
The above displays a WebView and a progress bar indicating the loading progress of the web page. First, we initialize WebViewController class, which is used to manage the WebView.
In the initState() method of _MyStatefulWidgetState, the WebViewController is set up with a NavigationDelegate. The NavigationDelegate listens for events like onPageStarted, onProgress, and onPageFinished, which allow the application to update the loading progress of the web page.
The loadRequest() method of the WebViewController is called to load a web page. The AppBar has a title and a refresh button that calls the reload() method of the WebViewController.
The body is a Stack widget that contains a WebViewWidget and a LinearProgressIndicator. The WebViewWidget is a custom widget that wraps the WebView widget and takes the WebViewController as an argument.
The LinearProgressIndicator is displayed only when the web page is still loading, and its value is set to the loadingPercentage, which is updated by the NavigationDelegate.
Following is the output.
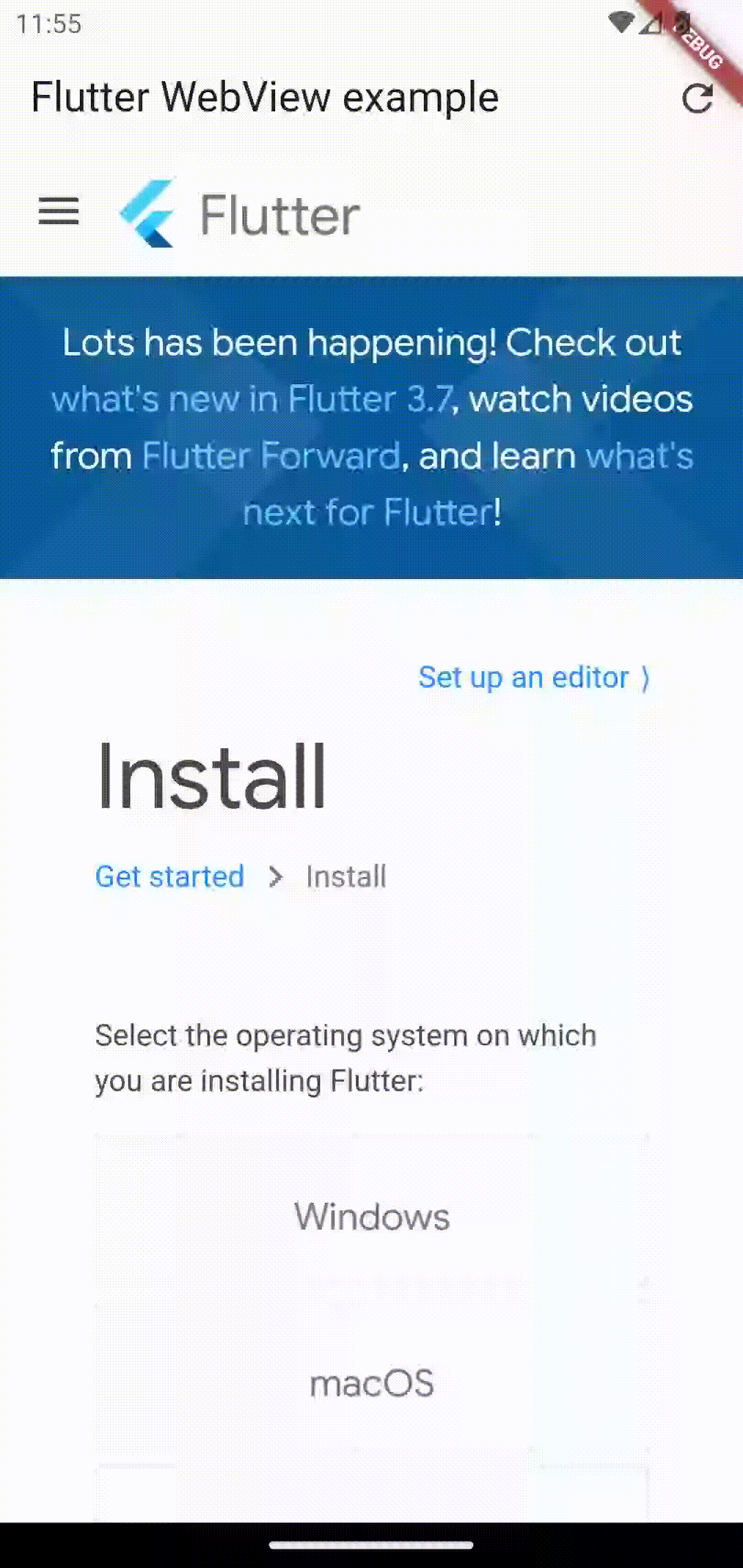
If you want to add a back button to the webview then see this: how to add webview with back button in flutter.
I hope this tutorial to reload Flutter WebView is helpful for you.