How to Make TextField Read Only in Flutter
I already have a blog post on how to disable Flutter TextField. In this tutorial, let’s learn how to make TextField read-only in Flutter.
You might be thinking what’s the difference between disabling and making read-only? When the TextField is made read-only then you cannot change the text but you can select the text. The property readOnly helps you in this case.
See the code snippet given below.
TextField(
readOnly: true,
controller: _controller..text = 'Default Value',
decoration: const InputDecoration(
border: OutlineInputBorder(),
),
onSubmitted: (String value) {
debugPrint(value);
},
)
And following is the output.
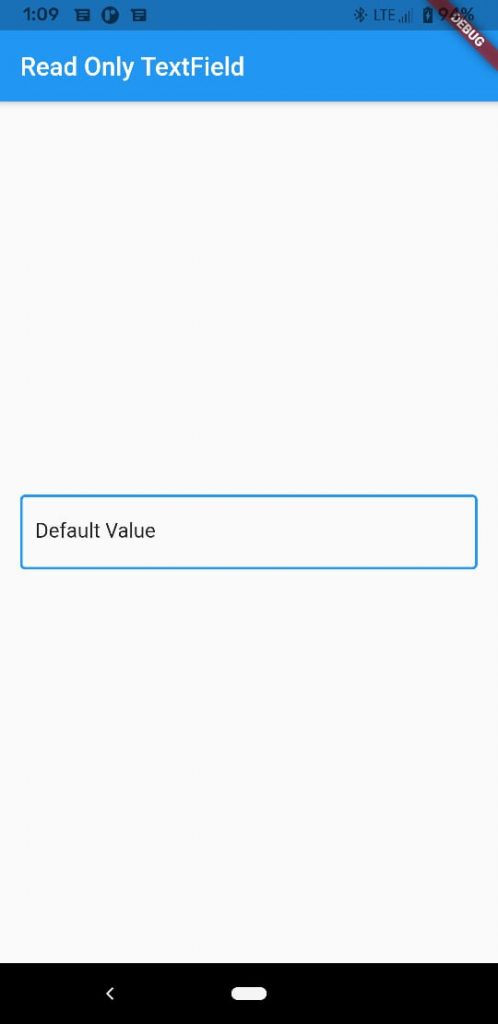
Following is the complete example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Border'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
readOnly: true,
controller: _controller..text = 'Default Value',
decoration: const InputDecoration(
border: OutlineInputBorder(),
),
onSubmitted: (String value) {
debugPrint(value);
},
),
));
}
}
That’s how you make TextField read-only in Flutter.