How to make Image Blur in Flutter
Image is one of the most used widgets in Flutter. Sometimes, we may want to blur the image to create visual effects. In this blog post, let’s learn how to make image blur in Flutter.
There is no direct property to change blur using the image widget. But you can use the ImageFiltered widget to create a blur effect in images. The image should be the child of the ImageFiltered widget.
See the following code snippet.
import 'dart:ui';
import 'package:flutter/material.dart';
ImageFiltered(
imageFilter: ImageFilter.blur(sigmaY: 5, sigmaX: 5),
child: Image.network(
'https://images.pexels.com/photos/6955476/pexels-photo-6955476.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2'),
)
The sigmaX and sigmaY help you control the blur effect to get the desired output. The output is given below.
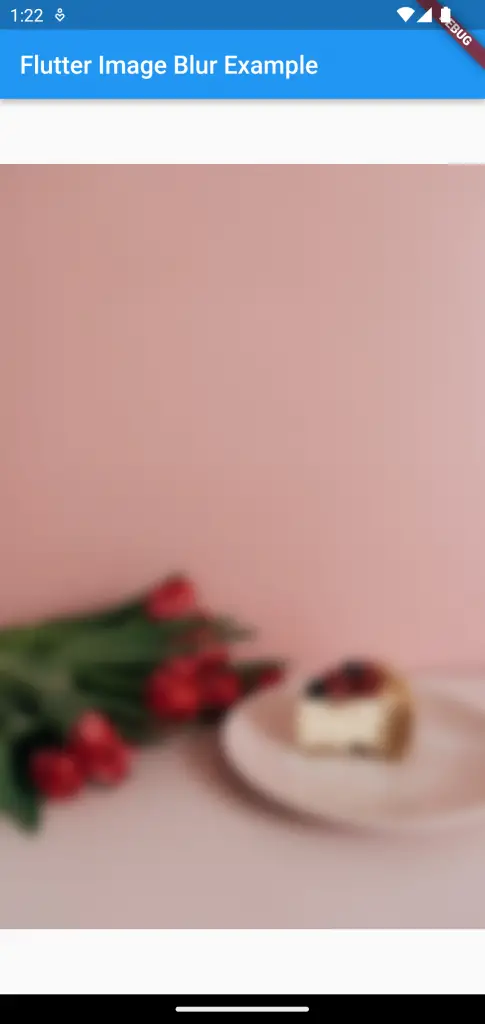
Following is the complete code for this Flutter image blur tutorial.
import 'dart:ui';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Image Blur Example'),
),
body: Center(
child: ImageFiltered(
imageFilter: ImageFilter.blur(sigmaY: 5, sigmaX: 5),
child: Image.network(
'https://images.pexels.com/photos/6955476/pexels-photo-6955476.jpeg?auto=compress&cs=tinysrgb&w=1260&h=750&dpr=2'),
)));
}
}
An alternative solution to creating image blur is to use BoxDecoration and BackdropFilter.
I hope this Flutter tutorial is helpful for you.