How to Make Container Clickable in Flutter
In normal cases, the Container widget doesn’t respond to touches. Sometimes, we may need to create custom widgets that are clickable. Let’s see how to make a container widget clickable widget in Flutter.
The GestureDetector widget is used to detect gestures. Hence, we use the GestureDetector in this example so that we can detect taps on the container. All you need is to wrap the Container widget with the GestureDetector.
See the complete code given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Clickable Container Example',
),
),
body: Center(
child: GestureDetector(
onTap: () {
const snackBar = SnackBar(content: Text("Clicked the Container!"));
ScaffoldMessenger.of(context).showSnackBar(snackBar);
},
child: Container(
padding: const EdgeInsets.all(12.0),
decoration: const BoxDecoration(
color: Colors.grey,
),
child: const Text('My Button'),
),
),
),
);
}
}
In the above Flutter example, when the container with the text ‘My Button’ is pressed we detect the tap and show the snack bar. Check the output of the example below.
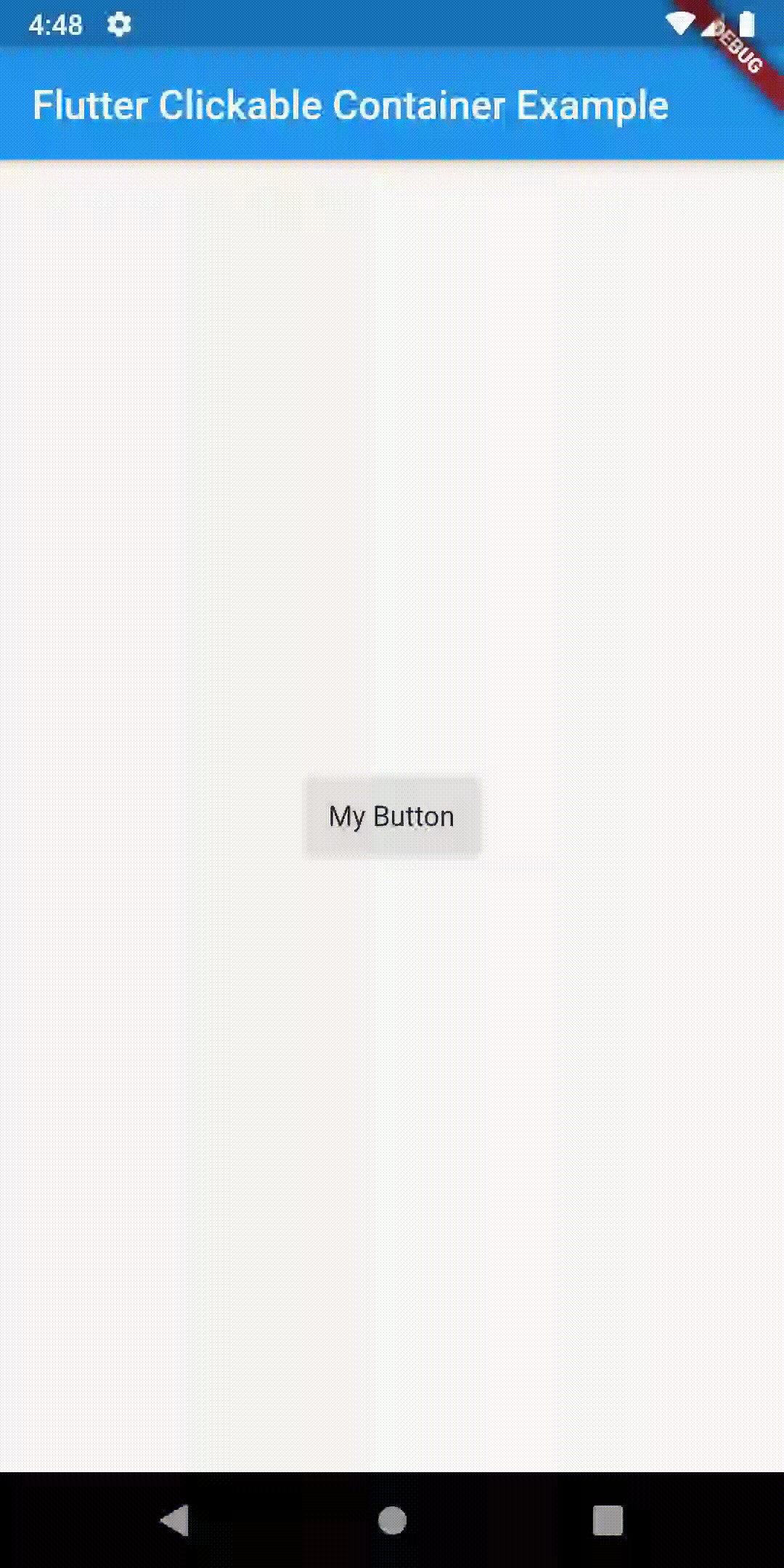
I hope this Flutter tutorial will help you!
I love the fact that after explanation and code samples there’s a screen video of the coded sample
I guess Inkwell also provide same result.