How to Get WebView Current URL in Flutter
The WebView is extremely useful to display a web page in Flutter. It also allows users to navigate through web pages. In this blog post, let’s learn how to get the current URL in Flutter.
We use webview_flutter Package in this tutorial. You can install it using the instructions given here.
If you want to retrieve the current URL being displayed in a WebView, you can use the WebViewController class. This class provides a method called currentUrl which returns a Future that resolves to the current URL.
See the code snippet given below.
getCurrentUrl() async {
var currentUrl = await controller.currentUrl();
debugPrint("Current URL: $currentUrl");
}
Most of the time, you may also want to know the URL when the webview changed by the user. In such cases, you can use onPageStarted of the NavigationDelegate.
WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
});
debugPrint('url: $url');
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
Following is the complete webview example where we show the current URL as Text.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late final WebViewController controller;
var loadingPercentage = 0;
var currentUrl = '';
@override
void initState() {
super.initState();
controller = WebViewController()
..setNavigationDelegate(NavigationDelegate(
onPageStarted: (url) {
setState(() {
loadingPercentage = 0;
currentUrl = url;
});
debugPrint('url: $url');
},
onProgress: (progress) {
setState(() {
loadingPercentage = progress;
});
},
onPageFinished: (url) {
setState(() {
loadingPercentage = 100;
});
},
))
..loadRequest(
Uri.parse('https://flutter.dev'),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter WebView example'),
),
body: Stack(
children: [
WebViewWidget(
controller: controller,
),
if (loadingPercentage < 100)
LinearProgressIndicator(
value: loadingPercentage / 100.0,
),
Text('Current URL: $currentUrl'),
],
));
}
}
You will get the following output. The text changes when the user navigates to another web page.
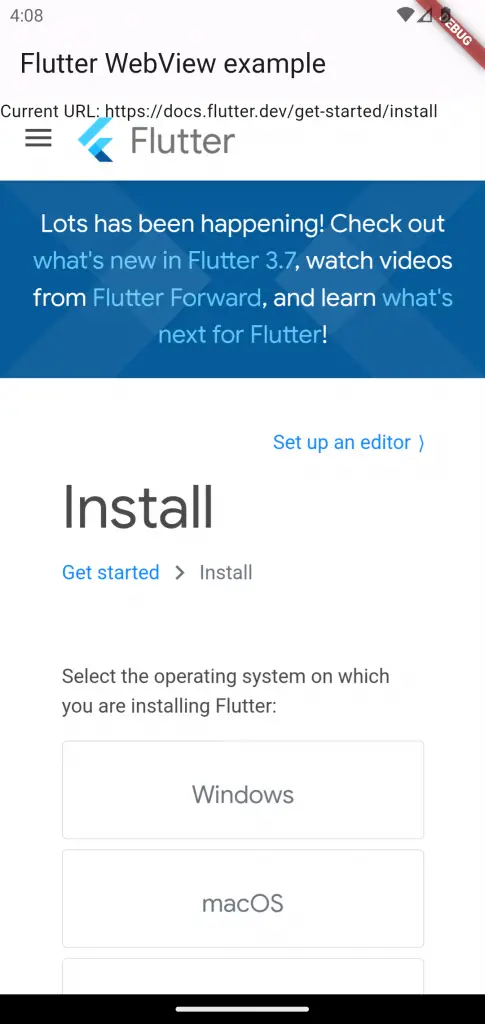
I hope this Flutter WebView tutorial to get the current URL is helpful for you.