How to Get Current Date in Flutter
Everyone knows the importance of dates and time in mobile apps. In this blog post, let’s learn how to get the current date in Flutter.
There’s a DateTime class in Dart that can be used to get the current date and time.
var now = new DateTime.now();
This will give us the output as follows.
2020-10-18 20:39:17.566238
Most of the time we want to show the current date in a specific format. You can convert DateTime to different formats using the Intl package.
Install the Intl package using the following command.
flutter pub add intl
You just need to use DateFormat class as given below.
import 'package:intl/intl.dart';
String now = DateFormat("yyyy-MM-dd hh:mm:ss").format(DateTime.now());
You will get the output as given below.
2020-10-18 09:01:08
As you only want the current date you can modify the format as given below.
import 'package:intl/intl.dart';
String now = DateFormat("yyyy-MM-dd").format(DateTime.now());
You will get the output in the following format.
2020-10-18
Following is the flutter example where I show the current date as Text.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyHomePage());
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Current Date Example',
),
),
body: Center(
child: Text(DateFormat("MM-dd-yyyy").format(DateTime.now()))));
}
}
Following is the output of the flutter current date example.
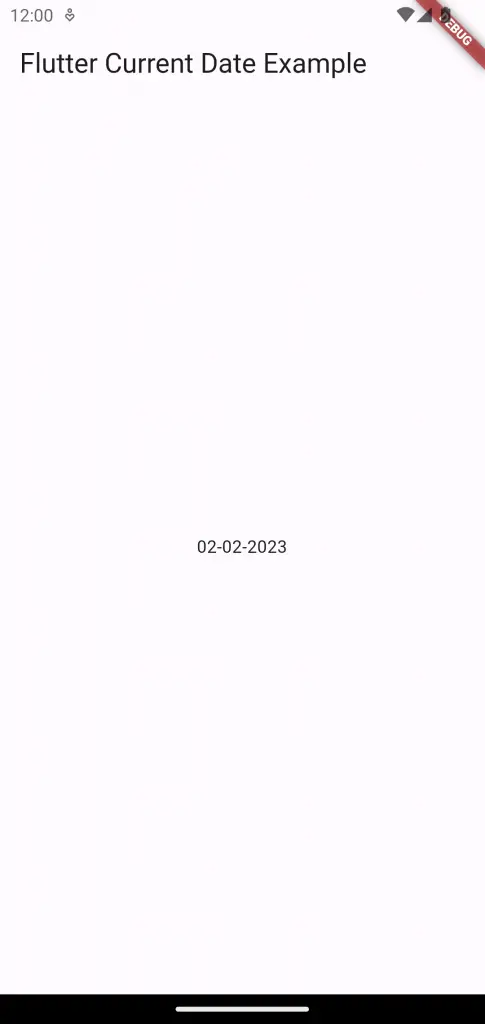
If you want to know how to add a date picker in Flutter then you can check my other tutorial here.
That’s how you show the current date in Flutter. I hope this tutorial is helpful for you.
One Comment