How to display Animated Gif in Flutter
Sometimes, you may want to add animated gifs instead of images to attract user attention through your mobile app. In this blog post, let’s learn how to display animated gifs in Flutter.
The Flutter Image widget is quite powerful. It supports various formats such as jpeg, png, gif, animated gif, bmp, etc. Yes, we can use the same Image widget to show gifs too.
See the following code snippet.
Image.network(
'https://cdn.pixabay.com/animation/2022/12/05/15/23/15-23-06-837_512.gif',
width: 300,
height: 500)
Here, we show a gif from internet. You can also show gifs from assets in Flutter.
Following is the output.
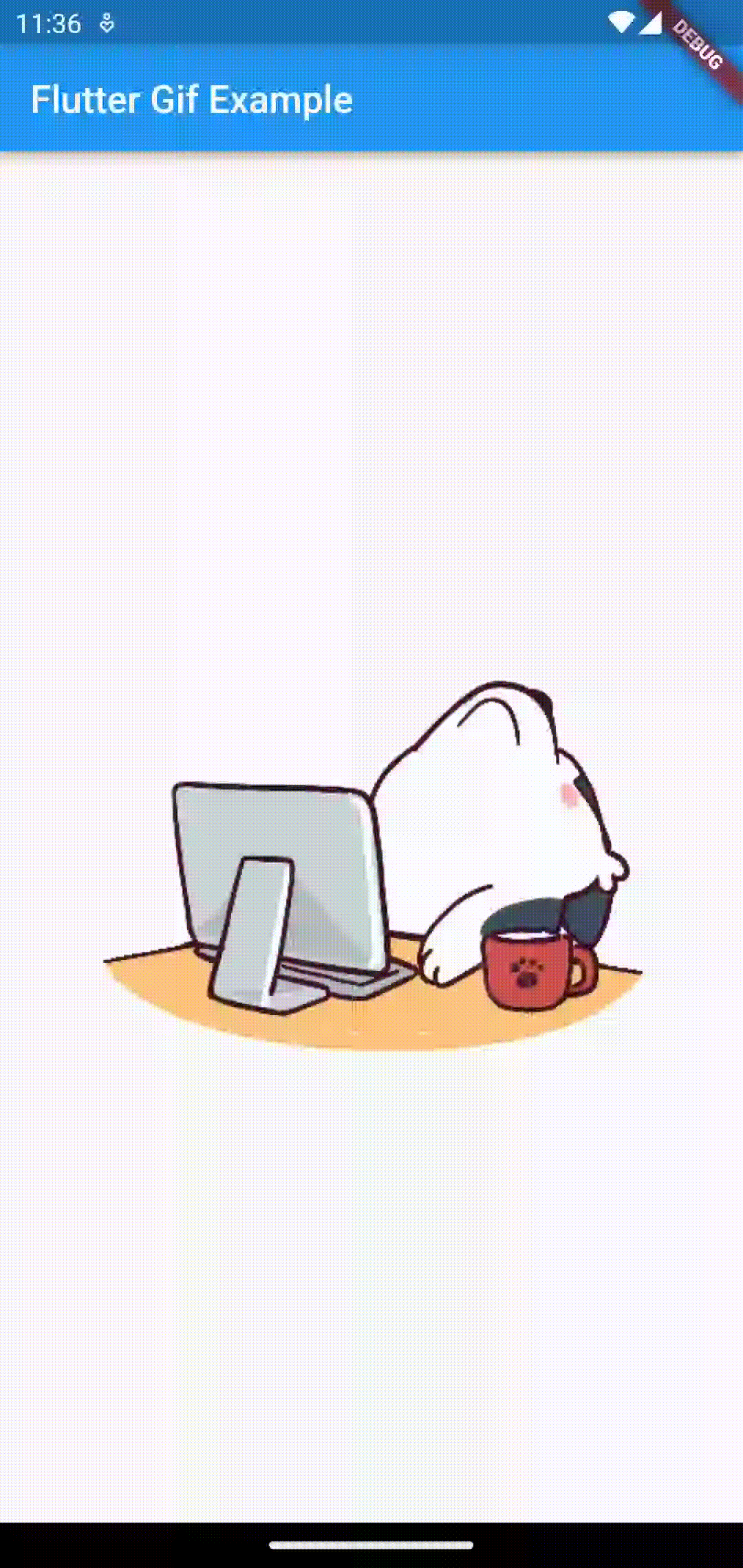
The complete code to display gif in Flutter is given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Gif Example'),
),
body: Center(
child: Image.network(
'https://cdn.pixabay.com/animation/2022/12/05/15/23/15-23-06-837_512.gif',
width: 300,
height: 500)));
}
}
That’s how you show gif in Flutter.