How to Disable TextButton in Flutter
Flutter has so many Button widgets and the TextButton is one of them. Sometimes, we may want to disable a button. In this Flutter tutorial, let us check how to disable a TextButton.
Disabling TextButton is pretty easy. All you need is to pass a null value to the onPressed property. See the code snippet given below.
TextButton(
style: TextButton.styleFrom(
padding: const EdgeInsets.all(10),
foregroundColor: Colors.red,
textStyle: const TextStyle(fontSize: 20)),
onPressed: null,
child: const Text('Disabled TextButton'),
),
The color of the TextButton becomes grey when it is disabled. The output is given below.
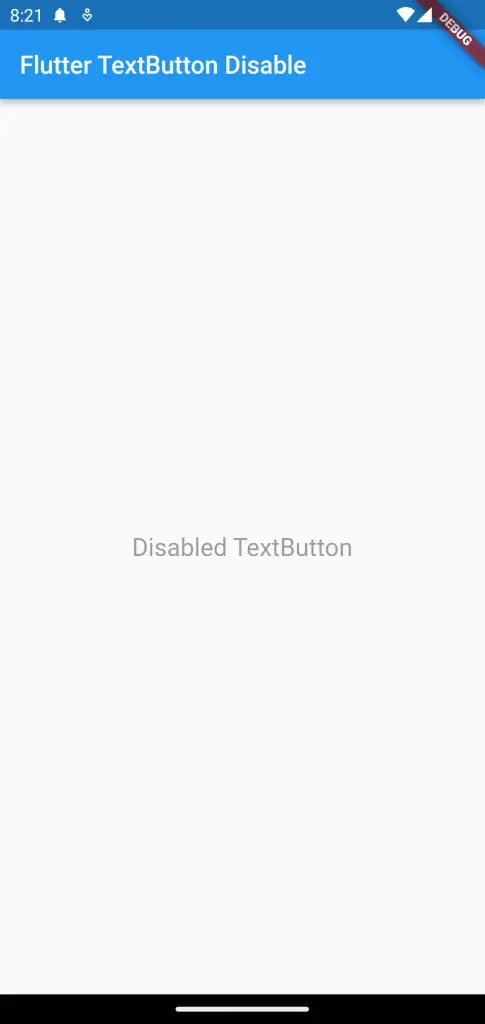
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter TextButton Disable',
),
),
body: Center(
child: TextButton(
style: TextButton.styleFrom(
padding: const EdgeInsets.all(10),
foregroundColor: Colors.red,
textStyle: const TextStyle(fontSize: 20)),
onPressed: null,
child: const Text('Disabled TextButton'),
),
));
}
}
That’s how you disable TextButton in Flutter.