How to Disable Click Events of a Screen in Flutter
In some special scenarios, we may want the user not to interact with the screen. In other words, we don’t want the clicks of users to get registered. Here, I am going to discuss how to disable click events of a screen in Flutter.
The click events of your mobile app screen can be absorbed by using the AbsorbPointer widget. With the property absorbing we can either disable or enable click-tap events.
In the following code, I have a ListView with two buttons.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Disable Clicks Example',
),
),
body: Center(
child: ListView(
children: <Widget>[
ElevatedButton(
child: const Text('Example 1'),
onPressed: () {},
),
ElevatedButton(
child: const Text('Example 2'),
onPressed: () {},
),
],
)));
}
}
Here, when we click on the buttons they respond by creating a ripple effect.
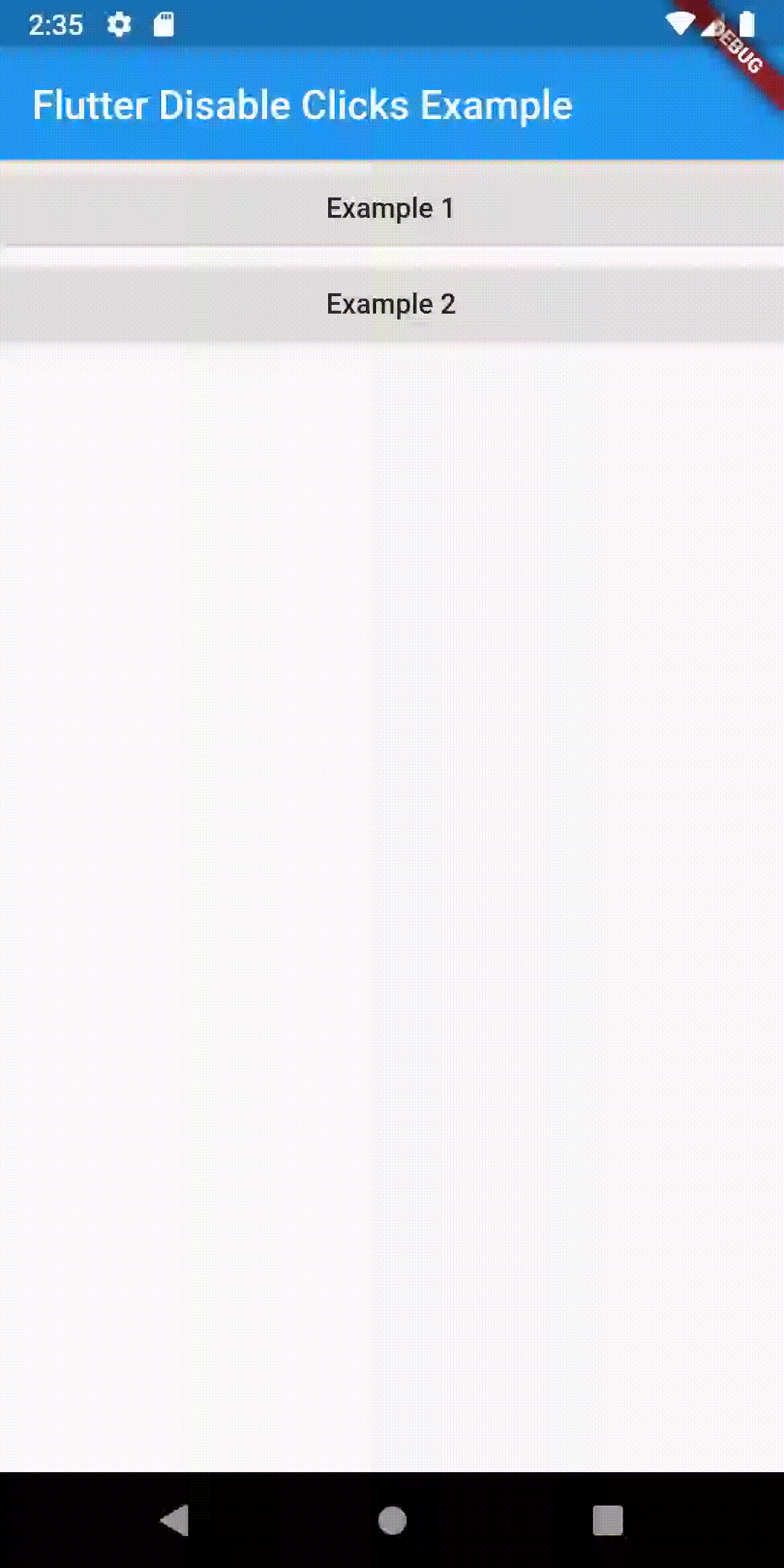
Now, let’s use the AbsorbPointer widget and make the value of the absorbing property true.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Disable Clicks Example',
),
),
body: AbsorbPointer(
absorbing: true,
child: Center(
child: ListView(
children: <Widget>[
ElevatedButton(
child: const Text('Example 1'),
onPressed: () {},
),
ElevatedButton(
child: const Text('Example 2'),
onPressed: () {},
),
],
)),
),
);
}
}
And now the buttons stop responding when we try to interact.
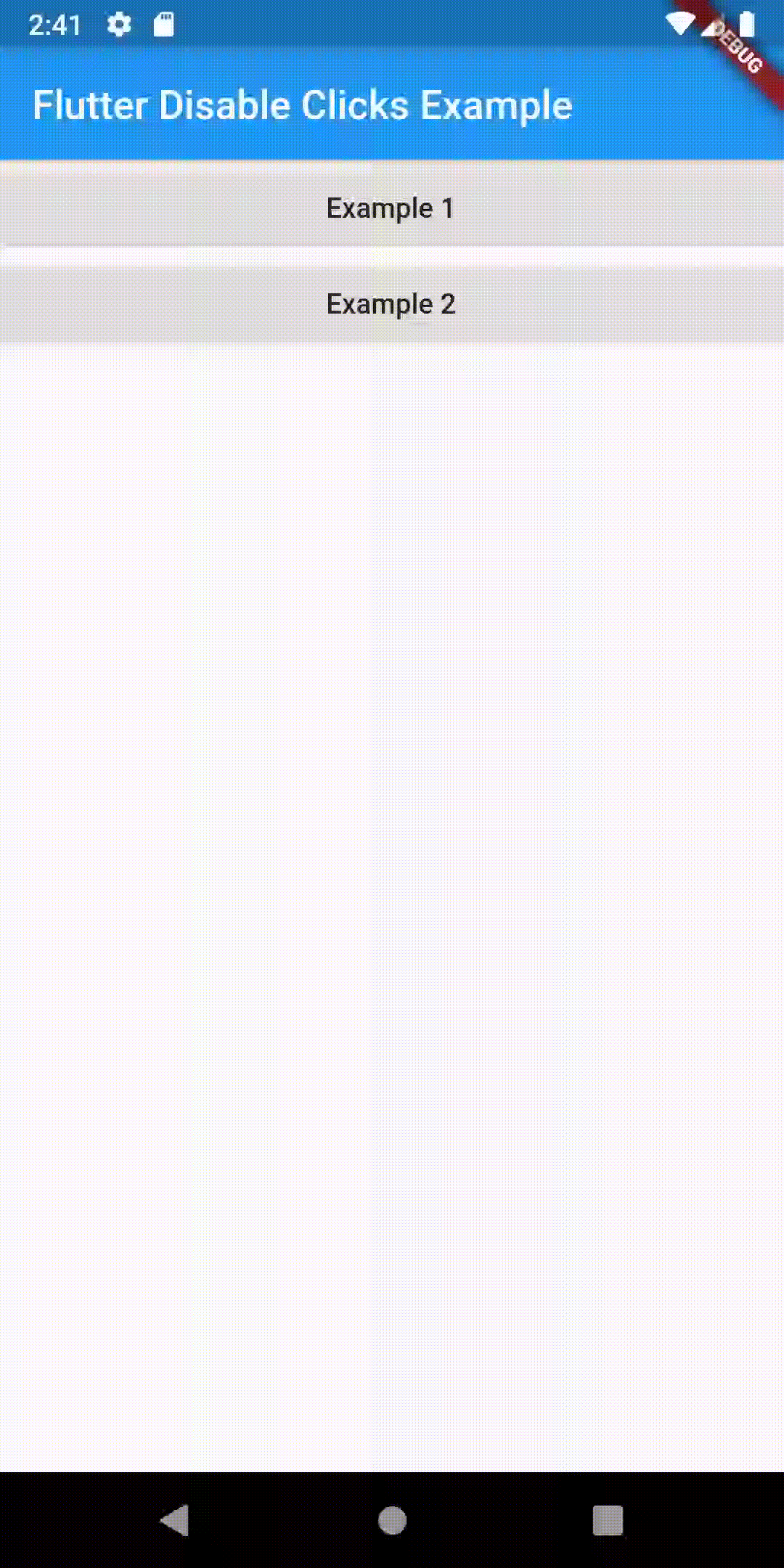
I hope this Flutter tutorial helped you.