How to Create ListView with Separator in Flutter
ListView makes its children scroll. Most of the time, we require a separator to distinguish between every child of ListView. This separator is basically a divider, which can be a line.
In the previous blog post, I wrote about how to use ListViews basically in Flutter. Now, let’s go through how to create a ListView with the divider in Flutter.
To create a divider with each of ListView child, you should use ListView.separated constructor. It accepts itemBuilder as well as separatorBuilder. The separator can be built using the Divider class. The Divider is basically used to draw thin horizontal lines.
See the snippet given below:
ListView.separated(
itemCount: data.length,
separatorBuilder: (BuildContext context, int index) => Divider( height: 3, color: Colors.white),
itemBuilder: (BuildContext context, int index) {
return Container(
height: 150,
color: Colors.red,
child: Center(child: Text(data[index])),
);
}
)
Following is the full example of Listview with separators in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
final data = [
"one",
"two",
"three",
"four",
"five",
"six",
"seven",
"eight",
"nine",
"ten"
];
MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter ListView with Separator Example',
),
),
body: ListView.separated(
itemCount: data.length,
separatorBuilder: (BuildContext context, int index) =>
const Divider(height: 3, color: Colors.white),
itemBuilder: (BuildContext context, int index) {
return Container(
height: 150,
color: Colors.red,
child: Center(child: Text(data[index])),
);
}));
}
}
And here’s the output:
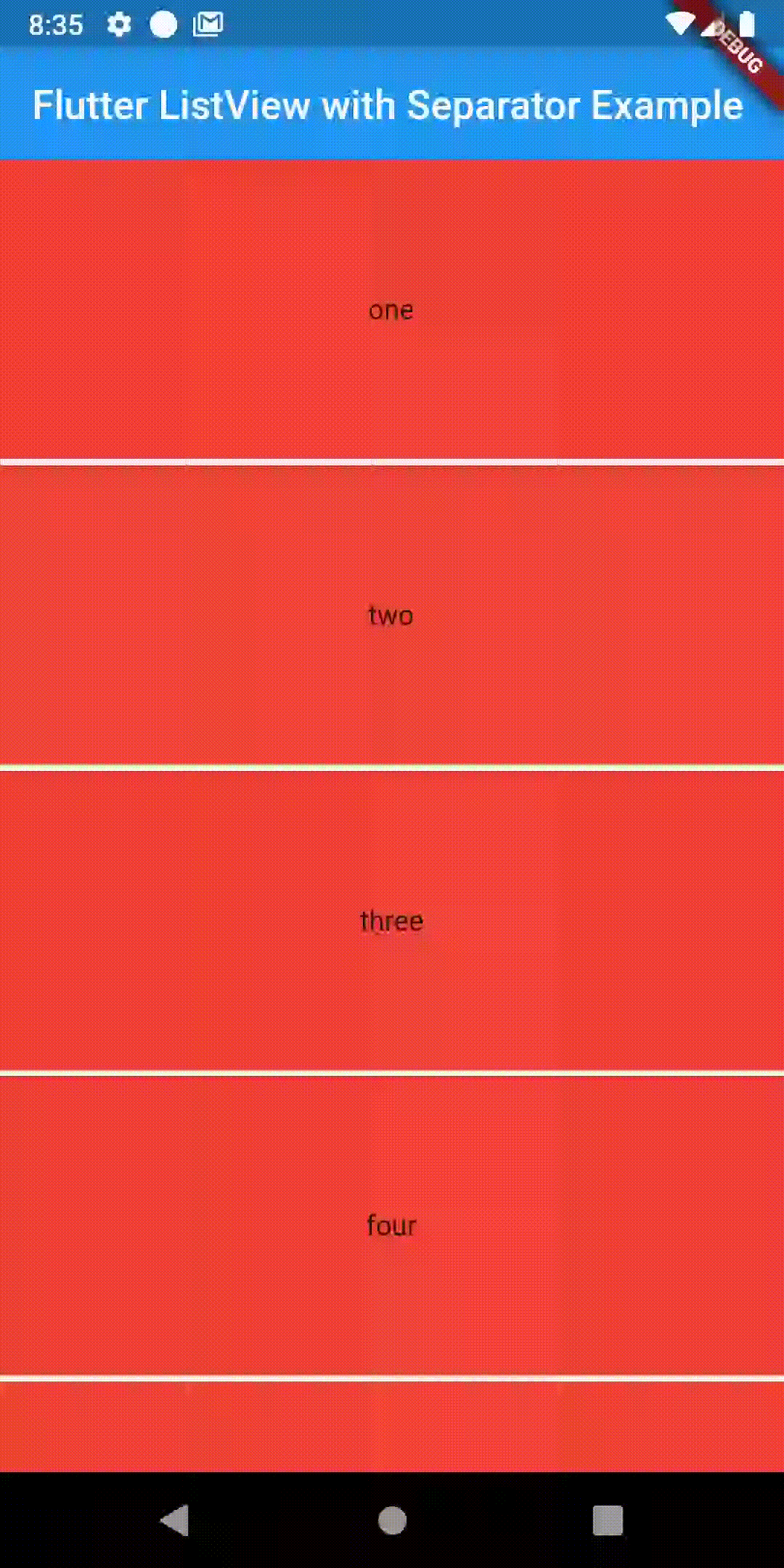
I hope this Flutter tutorial helped you! Thank you for reading!