How to Create Functions in Flutter
The popularity of Flutter is so high that many mobile app developers using other frameworks or languages want to try it. The developers migrating from one framework to another always have so many confusions. For example, if you are a react native developer and wanna try flutter then you have to learn more about Flutter in your own way.
As you know, the Dart language is the base of Flutter. Hence creating functions in Flutter is the same as creating functions in Dart. In this blog post, let’s check how to create and use functions in Flutter. I hope it will be helpful for those who are new to Flutter.
Simple Function in Flutter
You can create a simple basic function in Flutter as given below.
void printText (String text) {
print(text);
}
As you see void is what you return in the function, printText is the name of the function and the String text is the parameter. You can also ditch void and write the function as given below.
printText (String text) {
print(text);
}
If you are returning something through the function then you have to mention the data type before the function name.
String printText (String text) {
print(text);
return 'Hello $text';
}
You can call this function from your widget as given below.
printText('World')
Following is the complete example where we use the above function to show the text Hello World.
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
String printText (String text) {
print(text);
return 'Hello $text';
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Welcome to Flutter',
home: Scaffold(
appBar: AppBar(
title: Text('Welcome to Flutter'),
),
body: Center(
child: Text(printText('World')),
),
),
);
}
}
You get the following output when you run the above code.
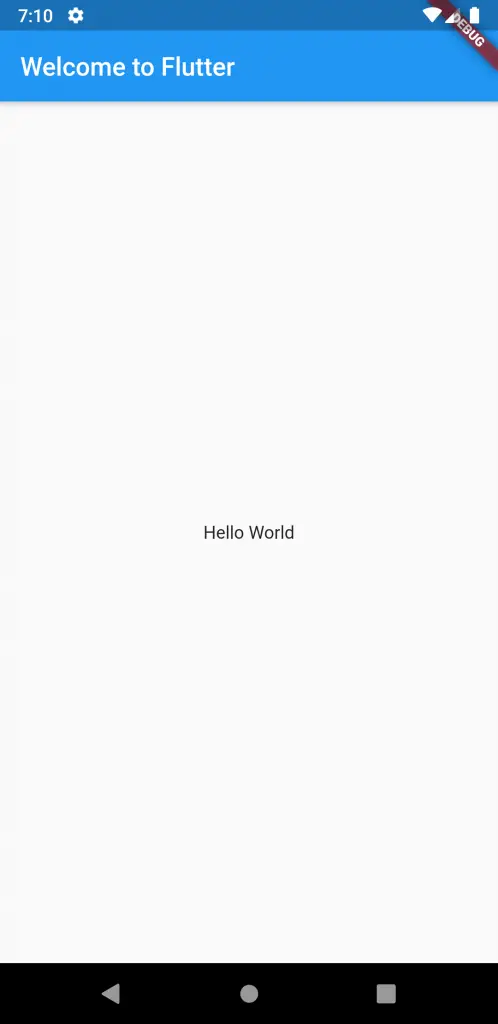
Arrow Function in Flutter
Are you a fan of arrow functions? Then you can use arrow functions in flutter too but only for single expression.
String printText (String text) => 'Hello $text';
I hope this basic tutorial on functions in Flutter is helpful for you.