How to Create Expanding and Collapsing Header in Flutter
Expanding and collapsing headers look beautiful when you have a list of things that the user scrolls through. Unlike other mobile app development platforms, creating expanding and collapsing headers in Flutter is easy.
We need the help of multiple widgets to create the header. As we are trying to create a scrolling effect we definitely need a CustomScrollView widget. We need the SliverAppBar widget which acts as the header of the list.
The FlexibleSpaceBar widget defines the area which expands, stretches, and collapses. The SliverList widget constitutes the remaining portion of the scroll view by providing children with a linear array.
Go through the following Flutter example code for more understanding.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return const Scaffold(body: MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
var data = [
'One',
'Two',
'Three',
'Four',
'Five',
'Six',
'Seven',
'Eight',
'Nine',
'Ten'
];
@override
Widget build(BuildContext context) {
return CustomScrollView(
slivers: <Widget>[
SliverAppBar(
pinned: true,
expandedHeight: 250.0,
flexibleSpace: FlexibleSpaceBar(
title: const Text('Collapsing Header'),
background: Image.network(
"https://cdn.pixabay.com/photo/2019/12/14/07/21/mountain-4694346_960_720.png",
fit: BoxFit.cover,
),
),
),
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return Container(
alignment: Alignment.center,
height: 100,
color: Colors.red[100],
child: Text(data[index]),
);
},
childCount: data.length,
),
),
],
);
}
}
And following is the output of this Flutter tutorial.
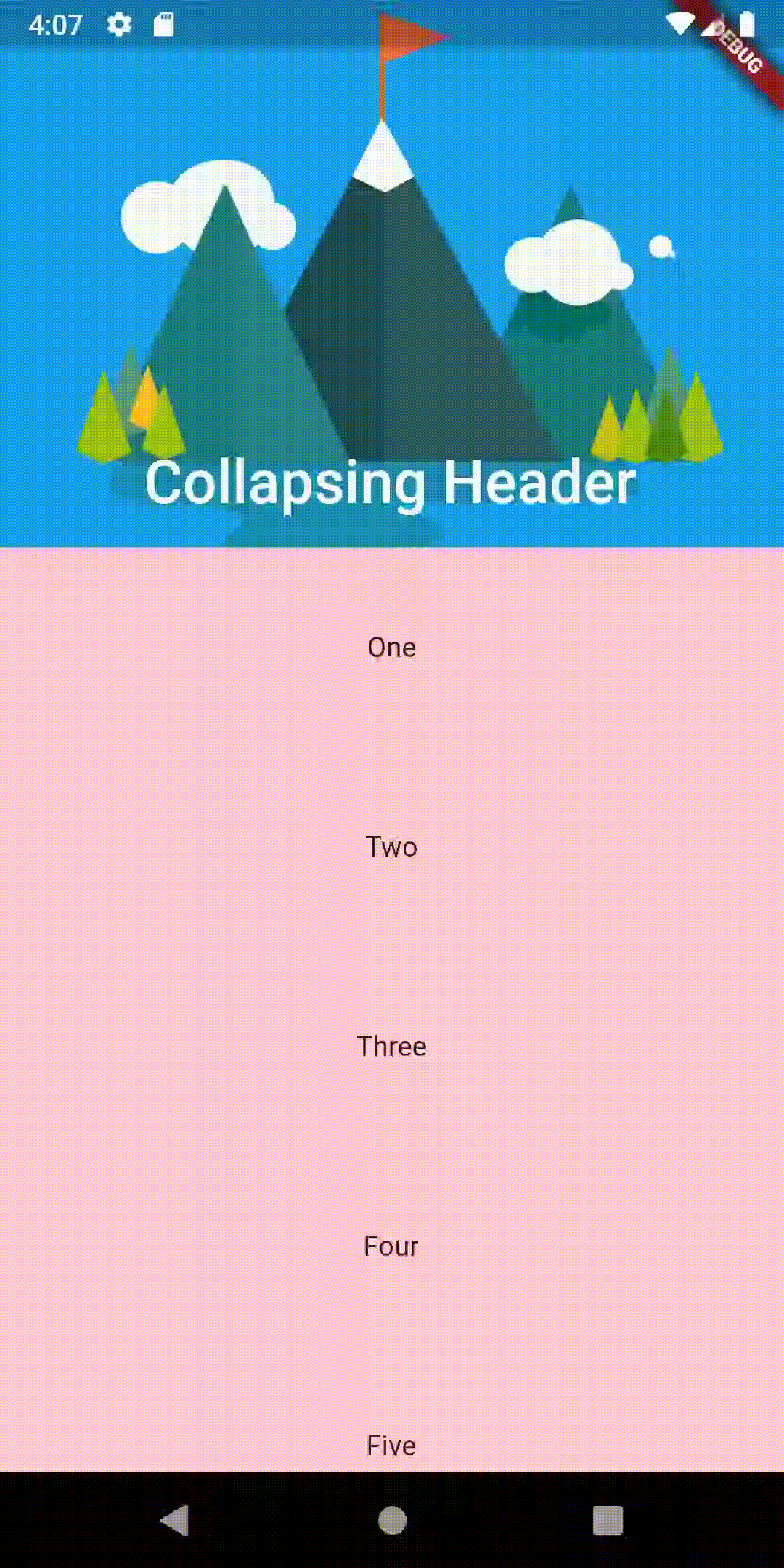
That’s how you add collapsing headers in Flutter.
I hope this flutter tutorial will help you to create a beautiful user interface.