How to Create DataTable with Search in Flutter
Having a search functionality in a data table can greatly enhance the user experience by allowing them to quickly and easily find specific information. In this blog post, let’s learn how to create a DataTable with search functionality in Flutter.
The DataTable widget is one of the most useful widgets in Flutter. It allows us to display a set of data in tabular format. You can create a search bar above the DataTable using the TextField widget.
Following is the complete code to display DataTable with a search bar.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: Scaffold(
appBar: AppBar(
title: const Text('Flutter DataTable Example'),
),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
List<dynamic> data = [
{"Name": "John", "Age": 28, "Role": "Senior Supervisor", "checked": false},
{"Name": "Jane", "Age": 32, "Role": "Administrator", "checked": false},
{"Name": "Mary", "Age": 28, "Role": "Manager", "checked": false},
{"Name": "Kumar", "Age": 32, "Role": "Administrator", "checked": false},
];
List<dynamic> filteredData = [];
final searchController = TextEditingController();
@override
void initState() {
filteredData = data;
super.initState();
}
@override
void dispose() {
searchController.dispose();
super.dispose();
}
void _onSearchTextChanged(String text) {
setState(() {
filteredData = text.isEmpty
? data
: data
.where((item) =>
item['Name'].toLowerCase().contains(text.toLowerCase()) ||
item['Role'].toLowerCase().contains(text.toLowerCase()))
.toList();
});
}
@override
Widget build(BuildContext context) {
return Column(children: [
Padding(
padding: const EdgeInsets.all(8.0),
child: TextField(
controller: searchController,
decoration: const InputDecoration(
hintText: 'Search...',
border: OutlineInputBorder(),
),
onChanged: _onSearchTextChanged,
),
),
SizedBox(
width: double.infinity,
child: DataTable(
columns: const <DataColumn>[
DataColumn(
label: Text('Name'),
),
DataColumn(
label: Text('Age'),
numeric: true,
),
DataColumn(
label: Text('Role'),
),
],
rows: List.generate(filteredData.length, (index) {
final item = filteredData[index];
return DataRow(
cells: [
DataCell(Text(item['Name'])),
DataCell(Text(item['Age'].toString())),
DataCell(Text(item['Role'])),
],
);
}),
),
),
]);
}
}
We have a sample data list of dictionaries that represent the rows in the table. The filteredData list is used to store the filtered data that is shown to the user in the table.
The searchController is used to manage the search text entered by the user. The initState method initializes the filteredData list to the entire data list, so initially, all the data is shown. The dispose method disposes of the searchController when the widget is no longer used.
The _onSearchTextChanged method filters the data list based on the text entered by the user in the search field. If the text is empty, it shows the entire data list, otherwise, it only shows the rows that contain either the name or the role of the row that matches the text entered.
The DataTable has columns for the name, age, and role of each item, and the rows are generated from the filteredData list.
You get the following output.
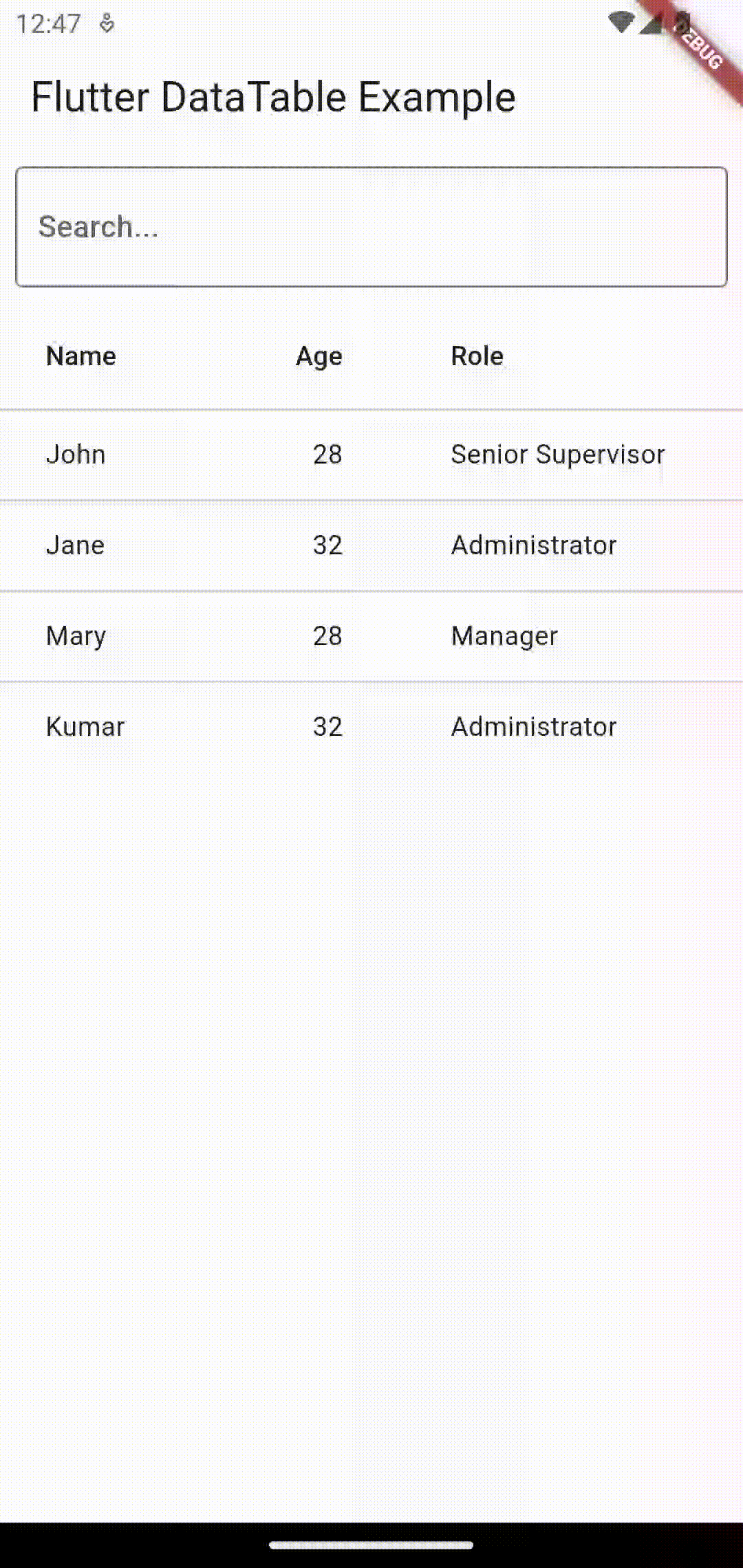
I hope this tutorial on Flutter DataTable search is helpful for you.