How to create Bordered Text in Flutter
Styling text is an important part of designing the UI of a mobile app. Sometimes, you may want to create bordered text to show colorful and elegant headlines. Let’s learn how to create bordered text in Flutter.
The Paint class in Flutter helps to draw on Canvas. By combining the Paint class and TextStyle we can obtain the desired results. See the code snippet given below.
Text(
'Bordered Text!',
style: TextStyle(
fontSize: 40,
foreground: Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1
..color = Colors.red,
),
)
The following is the outcome.
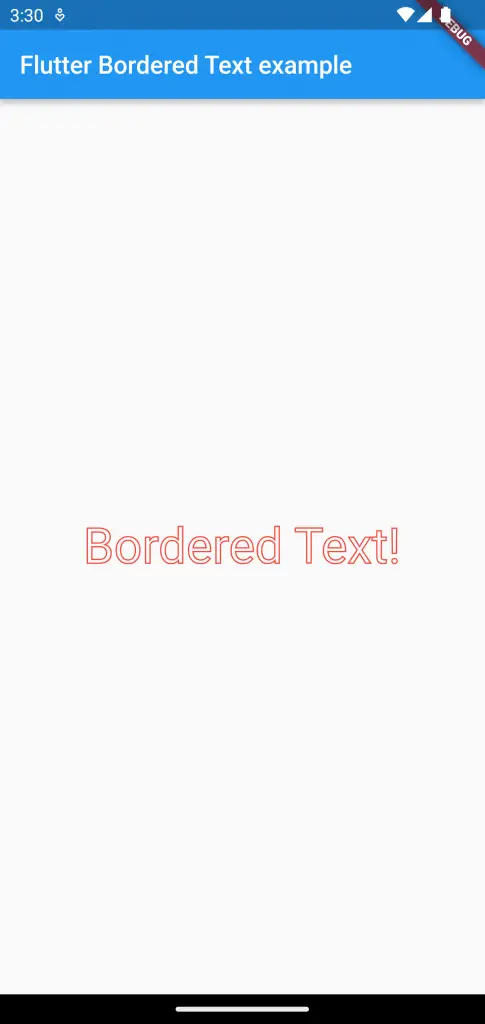
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Bordered Text example'),
),
body: Center(
child: Text(
'Bordered Text!',
style: TextStyle(
fontSize: 40,
foreground: Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1
..color = Colors.red,
),
),
),
);
}
}
That’s how you show bordered text in Flutter.