How to Create a Vertical Line in Flutter
Dividers are used to distinguish different components used in your mobile app. In this blog post, let’s check how to create a vertical divider or vertical line in Flutter.
We use the VerticalDivider widget to create a vertical line in Flutter. The VerticalDivider has useful properties such as width, thickness, color, indent, endindent, etc.
You can use it simply as given in the snippet below.
const VerticalDivider(
color: Colors.black,
thickness: 2,
width: 20,
indent: 200,
endIndent: 200,
)
Following is the complete example of using a vertical divider in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Vertical Divider Example',
),
),
body: Center(
child: Row(
children: <Widget>[
Container(
color: Colors.red,
height: 100,
width: 100,
),
const VerticalDivider(
color: Colors.black,
thickness: 2,
width: 20,
indent: 200,
endIndent: 200,
),
Container(
color: Colors.blue,
height: 100,
width: 100,
),
],
),
),
);
}
}
In the above Flutter example, we use Row, Container, and VerticalDivider widgets to create a vertical line between two containers. See the output of the Flutter example below.
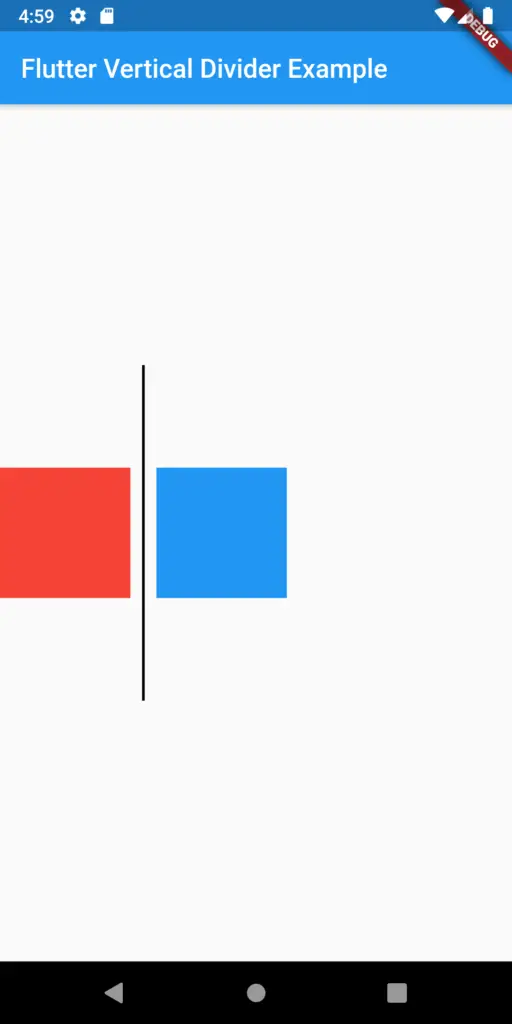
If you want to add a horizontal line, then see this Flutter tutorial.
I hope this flutter tutorial has helped you. Thank you for reading.