How to Create a PDF File in Flutter
PDF files are widely used for sharing and storing important documents, and with the rise of mobile applications, it’s become crucial to be able to generate PDF files on the go. In this blog post, let’s learn how to create a PDF in Flutter.
In this tutorial, we use the pdf package to generate a simple PDF file. The pdf package is a powerful plugin that has the capability to create multi-page documents with text, images, graphics, etc.
Install the package using the following command.
flutter pub add pdf
Also, you have to install the path_provider package for this tutorial.
flutter pub add path_provider
In order to preview and print the generated pdf you should install the printing package too.
flutter pub add printing
Then import these packages as given below.
import 'package:pdf/pdf.dart';
import 'package:pdf/widgets.dart' as pw;
import 'package:path_provider/path_provider.dart';
import 'package:printing/printing.dart';
See the following code snippet.
Future<Uint8List> generatePdf() async {
final pdf = pw.Document();
pdf.addPage(pw.Page(
pageFormat: PdfPageFormat.a4,
build: (pw.Context context) {
return pw.Center(
child: pw.Text(
"This is a sample pdf generated in Flutter",
style: const pw.TextStyle(
fontSize: 25,
),
),
); // Center
}));
var image = await networkImage('https://picsum.photos/250?image=9');
pdf.addPage(pw.Page(
pageFormat: PdfPageFormat.a4,
build: (pw.Context context) {
return pw.Center(
child: pw.Image(image),
); // Center
}));
final output = await getTemporaryDirectory();
debugPrint("${output.path}/example.pdf");
final file = File("${output.path}/example.pdf");
await file.writeAsBytes(await pdf.save());
return pdf.save();
}
It’s a sample function that creates a two-page PDF file. The first will have text whereas the second page will have an image. Then it is downloaded to the temporary directory.
Following is the complete code where you can preview the generated pdf.
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'dart:io';
import 'package:pdf/pdf.dart';
import 'package:pdf/widgets.dart' as pw;
import 'package:path_provider/path_provider.dart';
import 'package:printing/printing.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
Future<Uint8List> generatePdf() async {
final pdf = pw.Document();
pdf.addPage(pw.Page(
pageFormat: PdfPageFormat.a4,
build: (pw.Context context) {
return pw.Center(
child: pw.Text(
"This is a sample pdf generated in Flutter",
style: const pw.TextStyle(
fontSize: 25,
),
),
); // Center
}));
var image = await networkImage('https://picsum.photos/250?image=9');
pdf.addPage(pw.Page(
pageFormat: PdfPageFormat.a4,
build: (pw.Context context) {
return pw.Center(
child: pw.Image(image),
); // Center
}));
final output = await getTemporaryDirectory();
debugPrint("${output.path}/example.pdf");
final file = File("${output.path}/example.pdf");
await file.writeAsBytes(await pdf.save());
return pdf.save();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter PDF Example'),
),
body: PdfPreview(
build: (context) => generatePdf(),
),
);
}
}
You will get the following output.
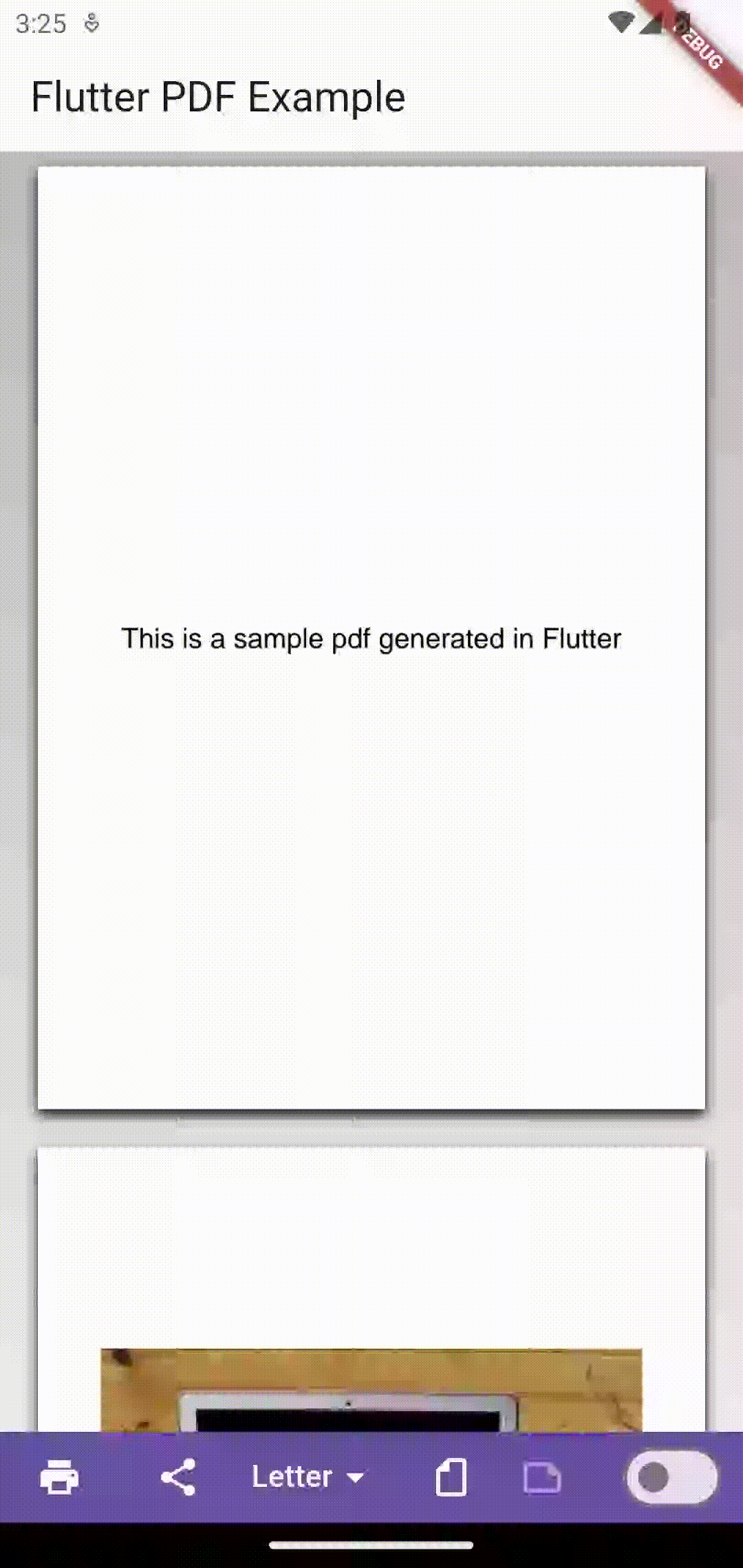
That’s how you create a PDF file in Flutter.