How to Change TextField Border Color in Flutter
I already have a blog post on how to add borders to TextField using OutlineInputBorder class. In this blog post, let’s check how to change the default color of TextField border in Flutter.
You can change the border color of your TextField using InputDecoration class, OutlineInputBorder class, and BorderSide class. See the code snippet given below.
TextField(
decoration: const InputDecoration(
focusedBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 2.0),
),
enabledBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.green, width: 2.0),
),
hintText: 'Enter your name',
),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
The border color is different for different states. When the TextField is focused, the green border color changes to red.
Following is the output.
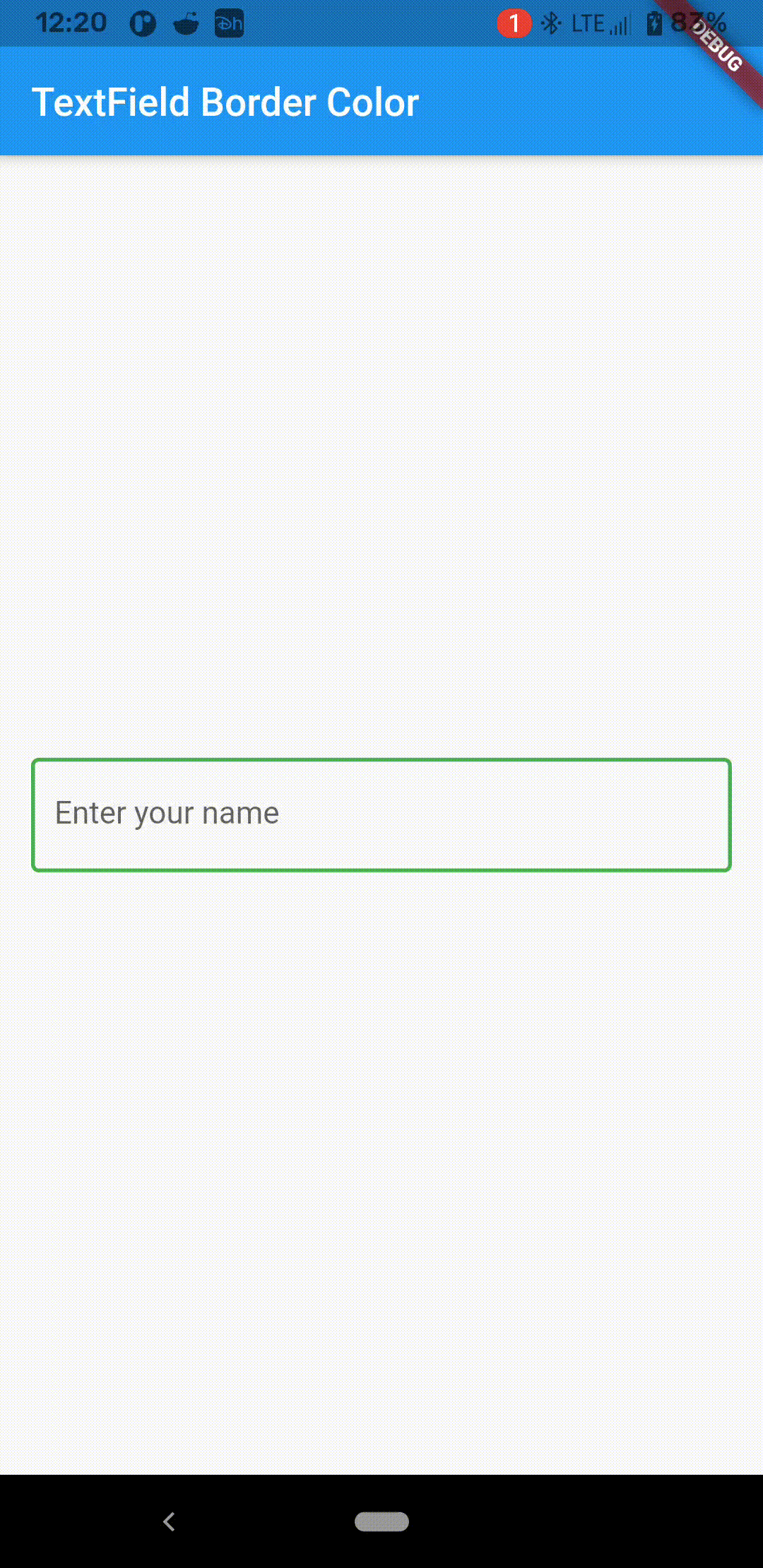
Following is the complete code for changing the TextField border color in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('TextField Border Color'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
decoration: const InputDecoration(
focusedBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 2.0),
),
enabledBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.green, width: 2.0),
),
hintText: 'Enter your name',
),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
));
}
}
That’s how you change the border color of TextField in Flutter.