How to change Text Opacity in Flutter
Opacity is an important aspect while designing mobile apps. Sometimes, you may want to change the opacity of the text instead of using a solid opaque color. Let’s learn how to change the Text opacity in Flutter.
The TextStyle class handles all the style aspects of the Text widget. With the help of Color.withOpacity method you can change the opacity of the Text color. See the following code snippet.
Text(
'This is Flutter Italic Text Tutorial!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 24, color: Colors.red.withOpacity(0.6)),
)
The value of opacity should be between 0.0 and 1.0. Following is the output of this Flutter text opacity tutorial.
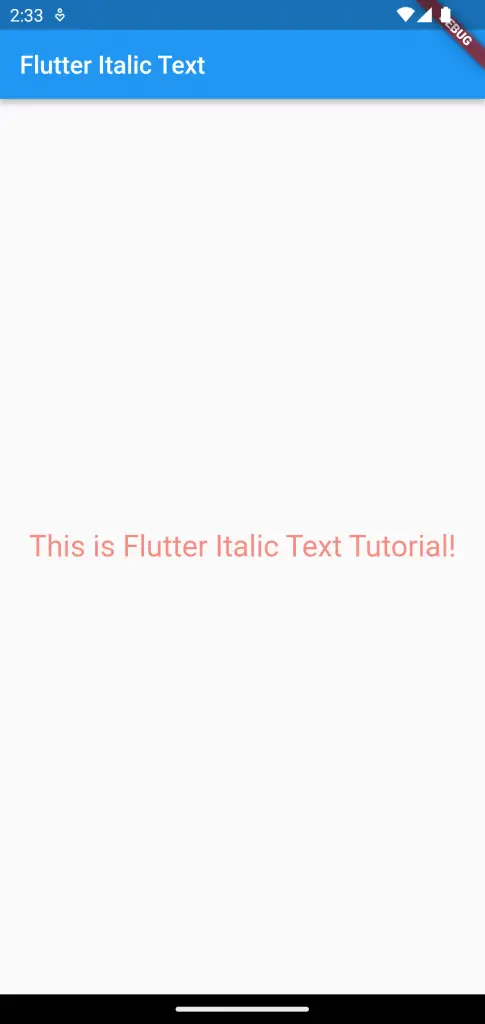
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Opacity'),
),
body: Center(
child: Text(
'This is Flutter Text Opacity Tutorial!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 24, color: Colors.red.withOpacity(0.6)),
)),
);
}
}
That’s how you change Text opacity in Flutter.