How to change Text Line Height in Flutter
A proper line height increases the readability of your app’s text content. In this Flutter tutorial, let’s see how to change the default line height of the Text widget.
The TextStyle class is used to stylize the Text widget in Flutter. The height property of the TextStyle class helps to set line height. See the following code snippet.
Text(
'This is a Flutter Text Line Height Tutorial! You can view the difference of line heights!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 18, height: 3),
)
The height is given a value of 3 which means the line height would be three times the size of the font. The output is given below.
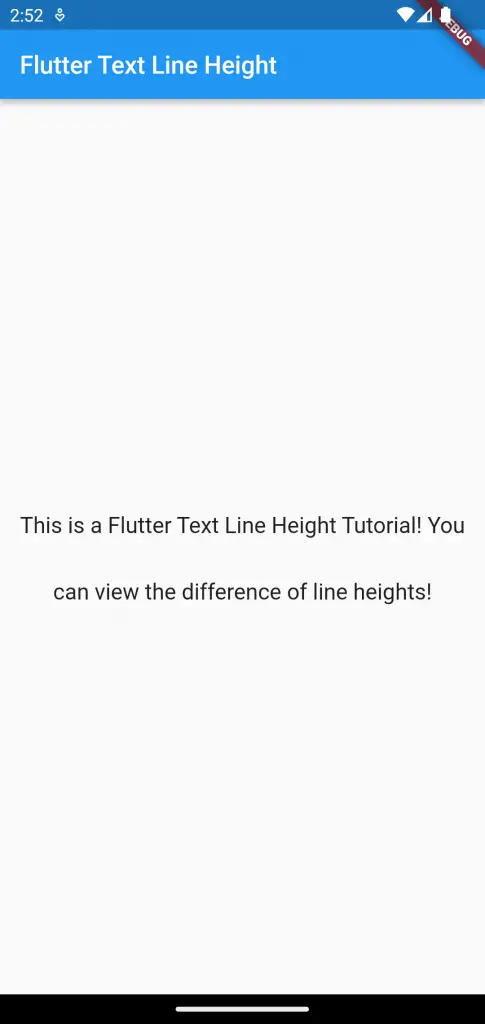
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Line Height'),
),
body: const Center(
child: Text(
'This is a Flutter Text Line Height Tutorial! You can view the difference of line heights!',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 18, height: 3),
)),
);
}
}
That’s how you change text line height in Flutter.