How to change Text Letter Spacing in Flutter
Styling text is an important part of mobile app UI design. Letter spacing helps to adjust the distance between each letter. Let’s check how to change the default letter spacing of Text in Flutter.
TextStyle class has a property named letterSpacing that helps to change the letter spacing of the Text. You have to specify the amount of space between each letter. You can even give negative value so that you can reduce the current letter spacing.
See the following code snippet.
Text('Letter spacing example!',
style: TextStyle(
fontSize: 18,
letterSpacing: 5,
)),
Following is the output.
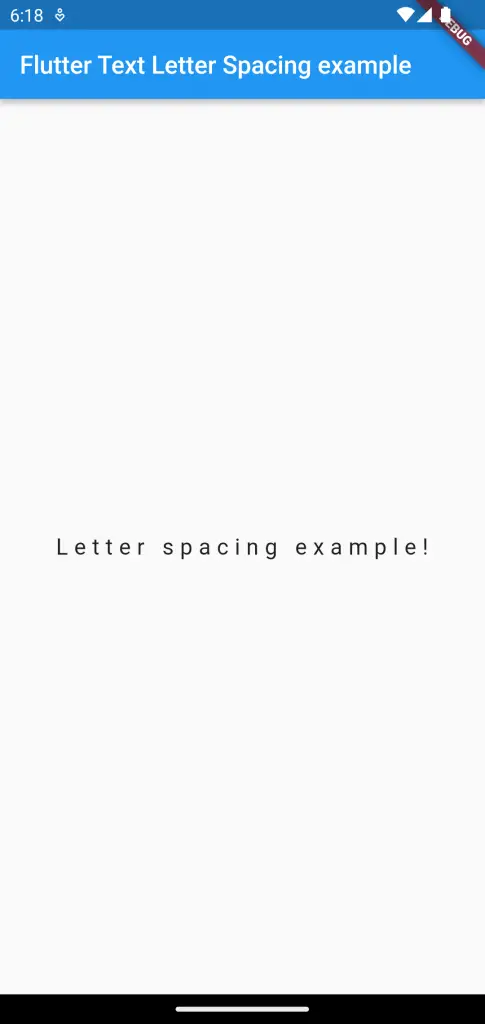
The complete code is given below.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Letter Spacing example'),
),
body: const Center(
child: Text('Letter spacing example!',
style: TextStyle(
fontSize: 18,
letterSpacing: 5,
)),
),
);
}
}
That’s how you change letter spacing in Flutter.