How to change Text Background Color in Flutter
As a mobile app developer, you may always want to style text properly to make the user interface elegant. In this Flutter tutorial, let’s learn how to set text background color easily.
Normally, we don’t play with the text background color. But in scenarios like highlighting text, having a bright background color is helpful. The TextStyle class has a property named backgroundColor to set a background color to the Text widget.
See the following code snippet.
Text('Flutter Text Background Color',
style: TextStyle(fontSize: 18, backgroundColor: Colors.yellow)),
),
Here, a yellow color background is applied to the text. Following is the output.
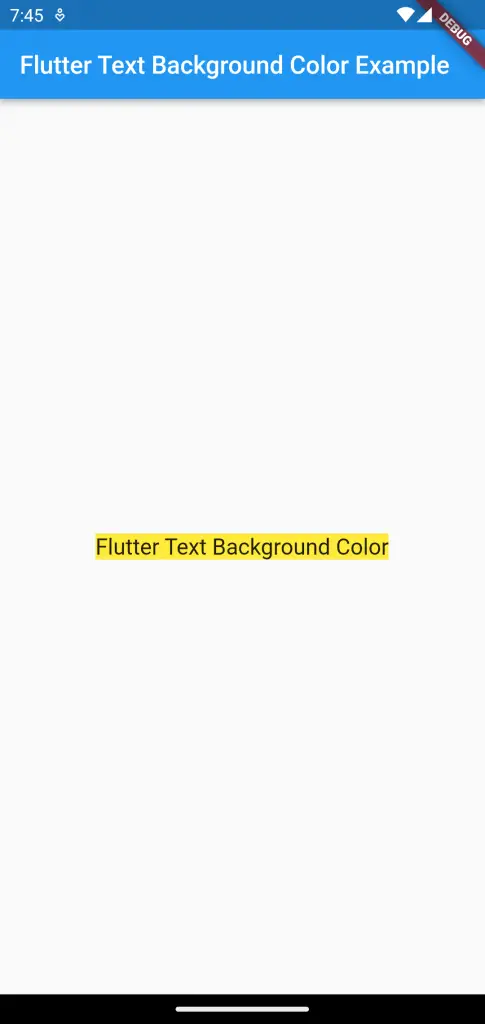
Following is the complete code for Flutter text background color tutorial.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Text Background Color Example'),
),
body: const Center(
child: Text('Flutter Text Background Color',
style: TextStyle(fontSize: 18, backgroundColor: Colors.yellow)),
),
);
}
}
That’s how you change text background color in Flutter. I hope this tutorial is helpful for you.