How to Change Placeholder Text Style of TextField in Flutter
The TextField is a very important widget that helps users to input text. Sometimes you may want to change the default placeholder style of TextField. In this Flutter tutorial, let’s check how to change the hintText style of the TextField widget.
Changing the hintText style is quite simple. You just need to use InputDecoration’s property named hintStyle.
See the code snippet given below.
TextField(
controller: _controller,
decoration: const InputDecoration(
hintText: 'Enter your name',
hintStyle: TextStyle(
fontSize: 30.0, color: Colors.red, fontWeight: FontWeight.bold),
),
onSubmitted: (String value) {
debugPrint(value);
},
),
Following is the output.
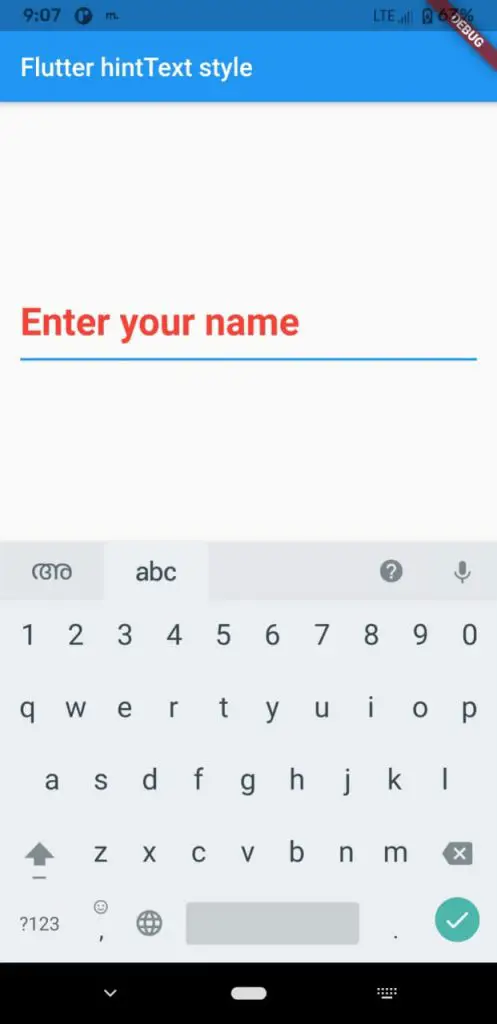
Following is the complete example.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter hintText style'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
controller: _controller,
decoration: const InputDecoration(
hintText: 'Enter your name',
hintStyle: TextStyle(
fontSize: 30.0, color: Colors.red, fontWeight: FontWeight.bold),
),
onSubmitted: (String value) {
debugPrint(value);
},
),
));
}
}
That’s how you change placeholder text style in Flutter.