How to change OutlinedButton Border Radius in Flutter
Rounded corners always make UI elements elegant. Tweaking border radius helps to create rounded corners. In this blog post, let’s learn how to change the OutlinedButton border radius in Flutter.
The OutlinedButton.styleFrom method helps to customize the style of the OutlinedButton. Using the shape property and the RoundedRectangleBorder class you can easily change border radius and make the corners rounded.
See the code snippet given below.
OutlinedButton.styleFrom(
side: const BorderSide(color: Colors.red, width: 2),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(30.0),
),
),
You will get the following output.
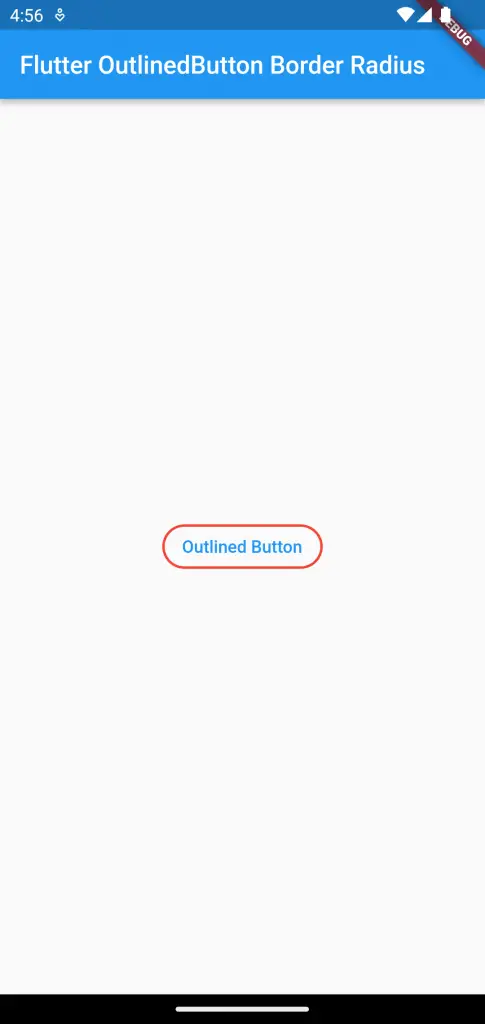
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter OutlinedButton Border Radius'),
),
body: Center(
child: OutlinedButton(
onPressed: () {},
style: OutlinedButton.styleFrom(
side: const BorderSide(color: Colors.red, width: 2),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(30.0),
),
),
child: const Text('Outlined Button'),
)));
}
}
That’s how you change border radius of the OutlinedButton in Flutter.