How to change Floating Action Button Splash Color in Flutter
The Floating Action Button (FAB) is one of the important components in material design. When the FAB is pressed A splash effect can be seen. In this blog post, let’s discuss how to change the Floating Action Button splash color in Flutter.
The FloatingActionButton widget has various properties to customize it. Using its splashColor property you can change the splash color easily.
See the code snippet given below.
FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.green,
splashColor: Colors.yellow,
child: const Icon(Icons.add),
),
Here, the color of FAB is green. When the button is pressed you can see the yellow color splash effect.
The output is given below.
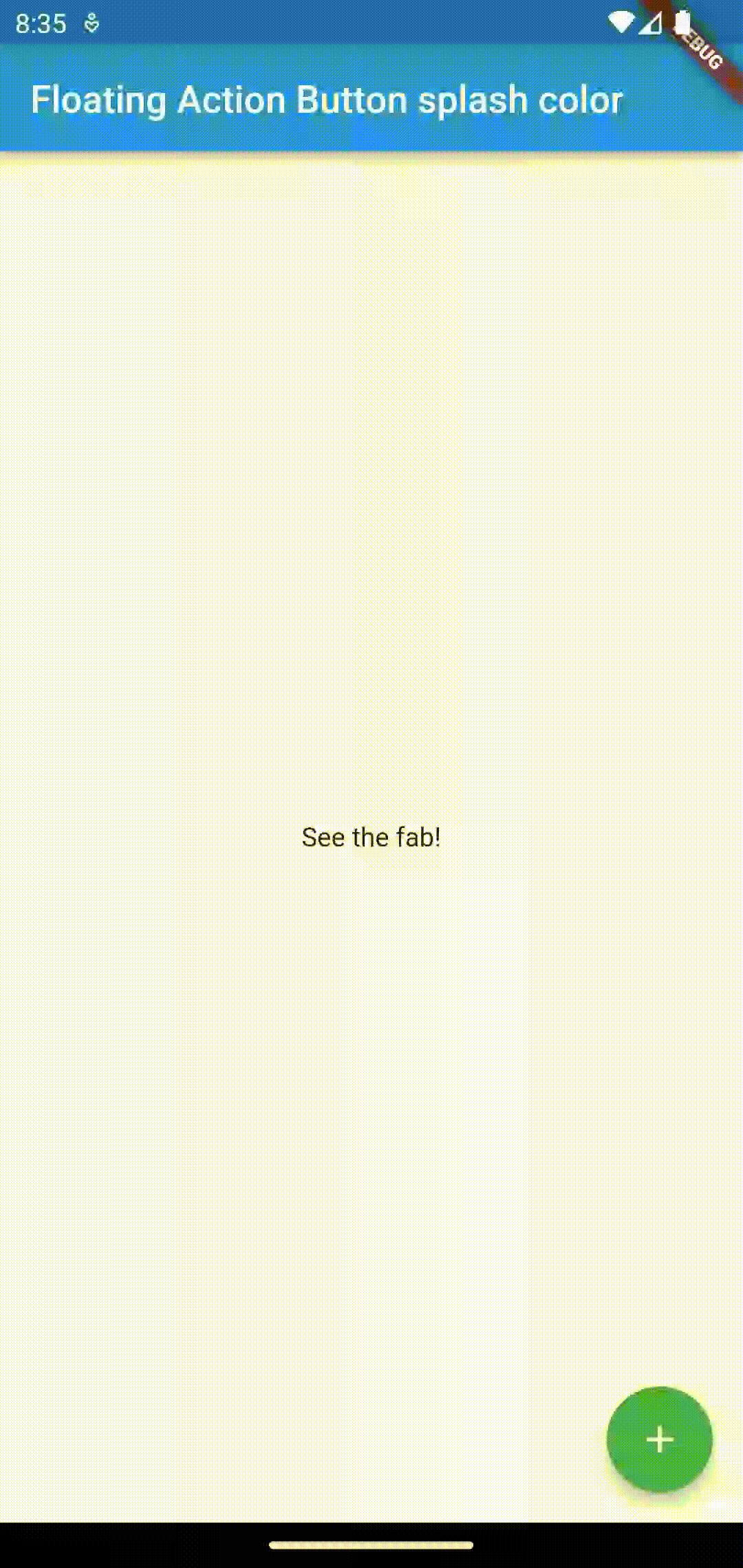
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Floating Action Button splash color'),
),
body: const Center(child: Text('See the fab!')),
floatingActionButton: FloatingActionButton(
onPressed: () {},
backgroundColor: Colors.green,
splashColor: Colors.yellow,
child: const Icon(Icons.add),
),
);
}
}
That’s how you change the Floating Action Button splash color in Flutter.