How to change Color of TextButton in Flutter
TextButton in Flutter helps to convert a text to button easily. The TextButton has different properties to customize it. In this Flutter tutorial, let’s learn how to change the color of TextButton.
By default, the color of TextButton is inherited from the theme. You can use styleFrom() method with TextButton to style the text, color, etc. See the following code snippet of the Flutter TextButton color.
TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.red,
textStyle: const TextStyle(fontSize: 20)),
onPressed: () {},
child: const Text('Flutter Text Button'),
),
You will get a red text button as output.
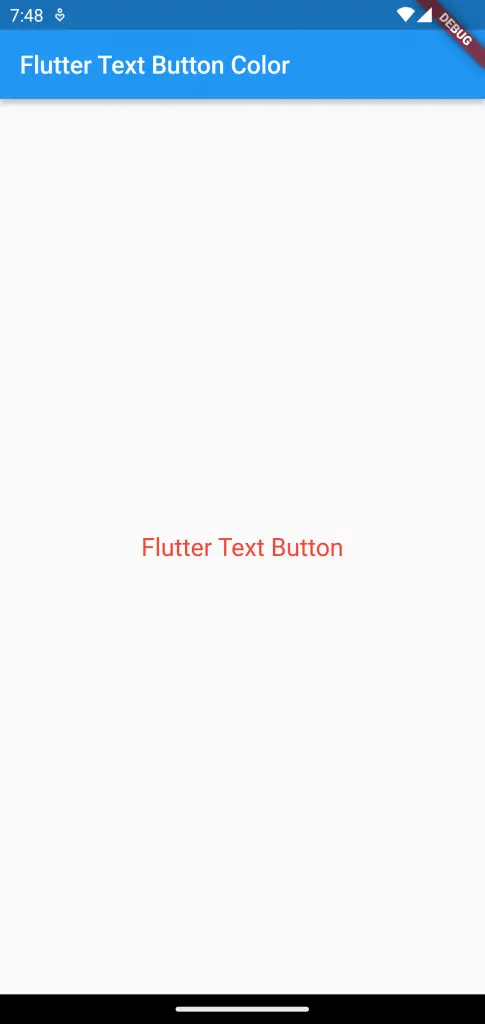
Following is the full code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Text Button Color',
),
),
body: Center(
child: TextButton(
style: TextButton.styleFrom(
foregroundColor: Colors.red,
textStyle: const TextStyle(fontSize: 20)),
onPressed: () {},
child: const Text('Flutter Text Button'),
),
));
}
}
I hope this Flutter TextButton color tutorial is helpful for you.