How to change Circular Progress Indicator Size in Flutter
The progress indicators are extremely useful UI components in a mobile app. In this blog post, let’s learn how to set the size for a circular progress indicator in Flutter.
We use the CircularProgressIndicator widget to add circular indicators in Flutter. But it doesn’t have a direct property to change the size. Hence, we should take help from other widgets for the desired result.
There are multiple solutions. One of them is to use the Transform class and scale the progress indicator according to your requirement.
See the code snippet given below.
Transform.scale(
scale: 2,
child: const CircularProgressIndicator(
backgroundColor: Colors.cyan,
color: Colors.red,
strokeWidth: 2,
))
Here, the size of the CircularProgressIndicator is made 2x of the default size. See the following output.
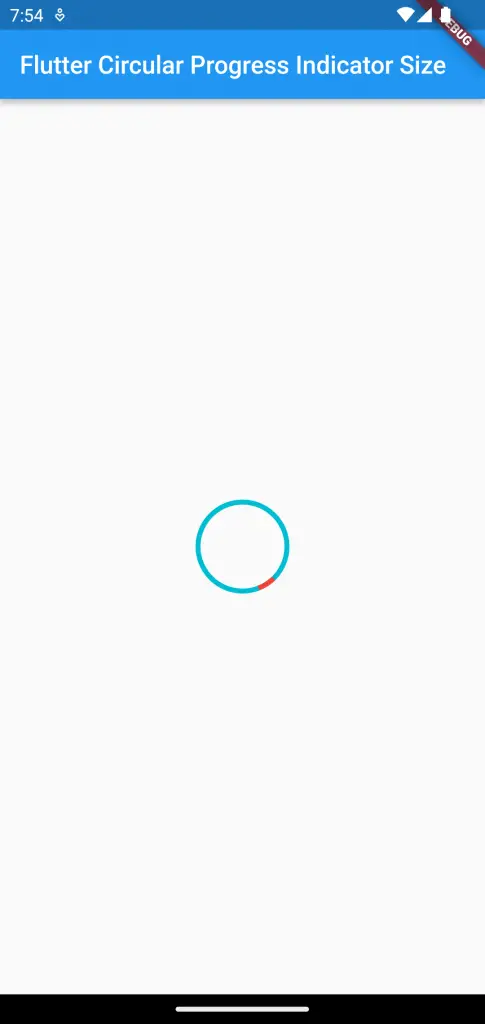
If you want to reduce the size, then you should choose a scale value of less than 1.
Transform.scale(
scale: 0.75,
child: const CircularProgressIndicator(
backgroundColor: Colors.cyan,
color: Colors.red,
strokeWidth: 2,
))
Then you will get the output given below.
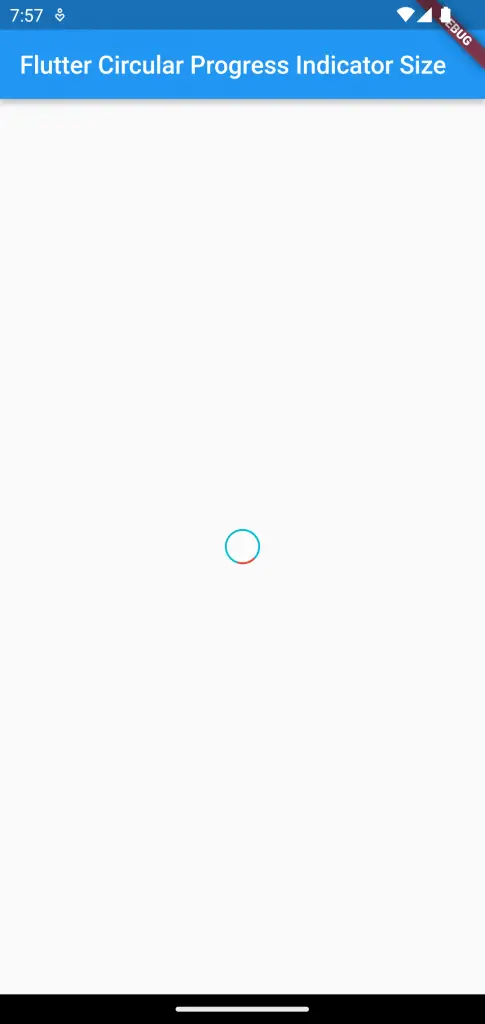
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Circular Progress Indicator Size'),
),
body: Center(
child: Transform.scale(
scale: 0.75,
child: const CircularProgressIndicator(
backgroundColor: Colors.cyan,
color: Colors.red,
strokeWidth: 2,
))));
}
}
That’s how you set the Flutter CircularProgressIndicator size.
You can also change the size of the linear progress indicator, see this Flutter tutorial.