How to change Checkbox Disabled Color in Flutter
The checkBox is an important UI component in mobile apps. In this blog post, let’s learn how to change the color of CheckBox when it is disabled.
You can disable CheckBox by making the onChanged property null. In order to change the color of CheckBox according to its state, we should use the fillColor property.
With the help of MaterialStateColor class, you can choose a custom color when the CheckBox is disabled. See the following code snippet.
Checkbox(
fillColor: MaterialStateColor.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return Colors.red;
}
return Colors.green;
},
),
value: checkBoxValue,
onChanged: null)
Here, the color of CheckBox when disabled is red. And for other states, the color would be green.
Following is the output.
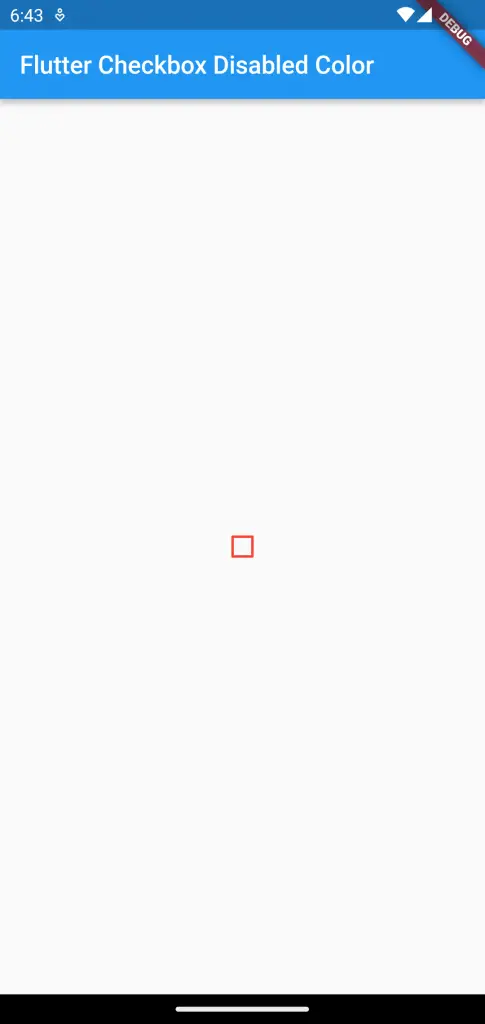
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Checkbox Disabled Color'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: Checkbox(
fillColor: MaterialStateColor.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return Colors.red;
}
return Colors.green;
},
),
value: checkBoxValue,
onChanged: null));
}
}
That’s how you change default disabled color of CheckBox in Flutter.