How to change Card Shadow Color in Flutter
The Card is an important component in Material UI design. In this blog post, let’s learn how to change the shadow color of a Card in Flutter.
The shadows around the Card widget are caused by the elevation. The Card has some useful properties and shadowColor is one among them. You can use the shadowColor to change the default color of shadows.
See the following code snippet.
Card(
margin: const EdgeInsets.all(20),
elevation: 10,
shadowColor: Colors.blue,
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
And you will get the output of card with blue color shadows.
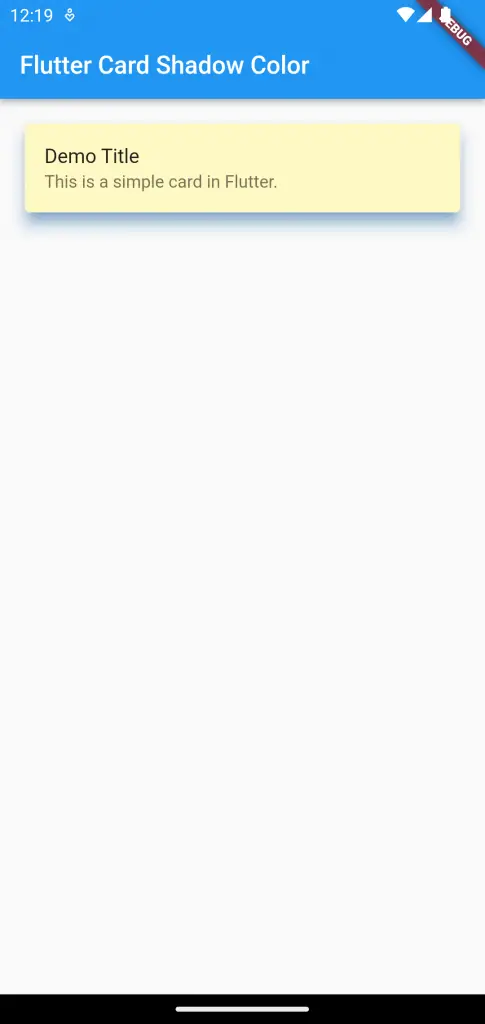
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card Shadow Color'),
),
body: Card(
margin: const EdgeInsets.all(20),
elevation: 10,
shadowColor: Colors.blue,
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
);
}
}
That’s how you change the Card shadow color in Flutter.