How to change AppBar Title Color in Flutter
AppBar is one of the important UI components of material design. In this short and simple Flutter tutorial, let’s check how to change the default AppBar title color.
The AppBar widget uses a Text widget to add the title. Hence, we can make use of the TextStyle class to change the color of the title. See the code snippet given below.
const Text(
'Flutter AppBar Text Color',
style: TextStyle(color: Colors.yellow),
),
),
A yellow color is given as the Text color. The output is given below.
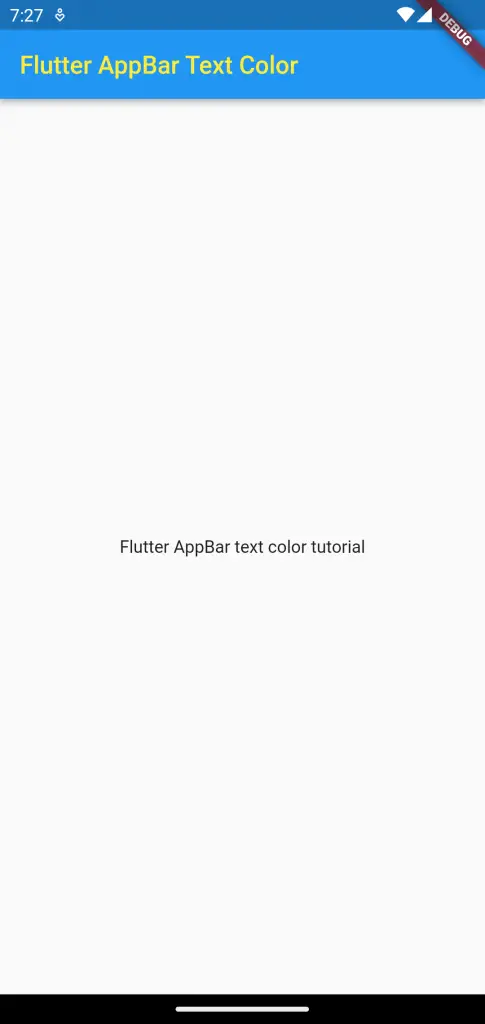
Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter AppBar Text Color',
style: TextStyle(color: Colors.yellow),
),
),
body: const Center(child: Text('Flutter AppBar text color tutorial')));
}
}
I hope you liked this Flutter AppBar text color tutorial.