How to change AppBar Elevation in Flutter
Everyone knows the importance of elevation in material design. An increase in elevation increases the shadow of the element. In this Flutter tutorial, let’s learn how to change the default elevation of AppBar.
AppBar has so many properties for customizing its content. One among them is elevation. You can specify the desired value to change the elevation distance. See the following code snippet.
AppBar(
title: const Text(
'Flutter AppBar Elevation',
),
elevation: 20,
),
Giving a value of 20 to elevation results in the following output.
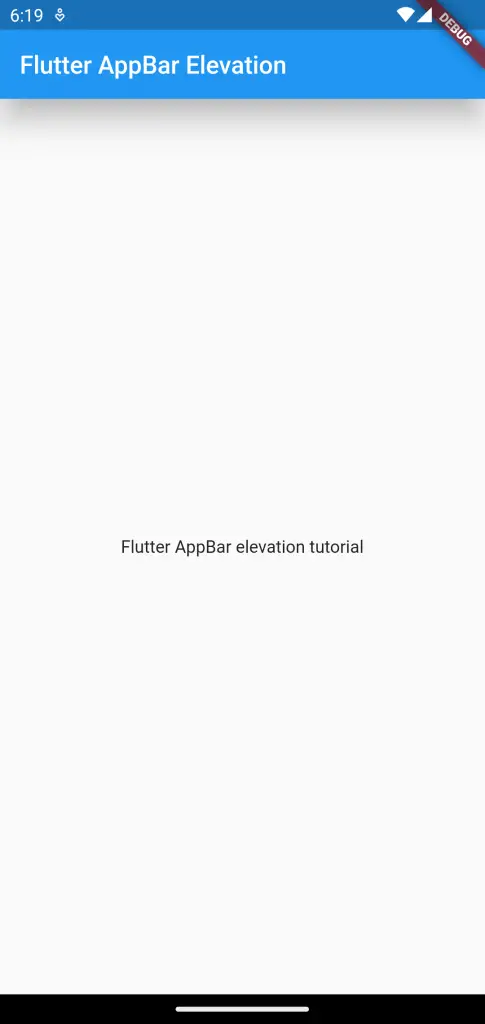
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter AppBar Elevation',
),
elevation: 20,
),
body: const Center(child: Text('Flutter AppBar elevation tutorial')));
}
}
That’s how you change Flutter AppBar elevation.