How to Arrange Children Widgets in Row and Column in Flutter
When we are building the user interface of a mobile app we always need to arrange each UI component in a particular manner. The Row and Column widgets are used to arrange children widgets in rows and columns in Flutter.
The Row widget is very simple to implement. You just need to wrap the Row widget and pass the children widgets as an array.
Row(
children: <Widget>[
Container(
color: Colors.red,
height: 100,
width: 100,
),
Container(
color: Colors.yellow,
height: 100,
width: 100,
),
Container(
color: Colors.blue,
height: 100,
width: 100,
),
],
),
Here’s the full example of the Flutter Row widget.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Row Example',
),
),
body: Center(
child: Row(
children: <Widget>[
Container(
color: Colors.red,
height: 100,
width: 100,
),
Container(
color: Colors.yellow,
height: 100,
width: 100,
),
Container(
color: Colors.blue,
height: 100,
width: 100,
),
],
),
),
);
}
}
And here’s the output of the flutter example.
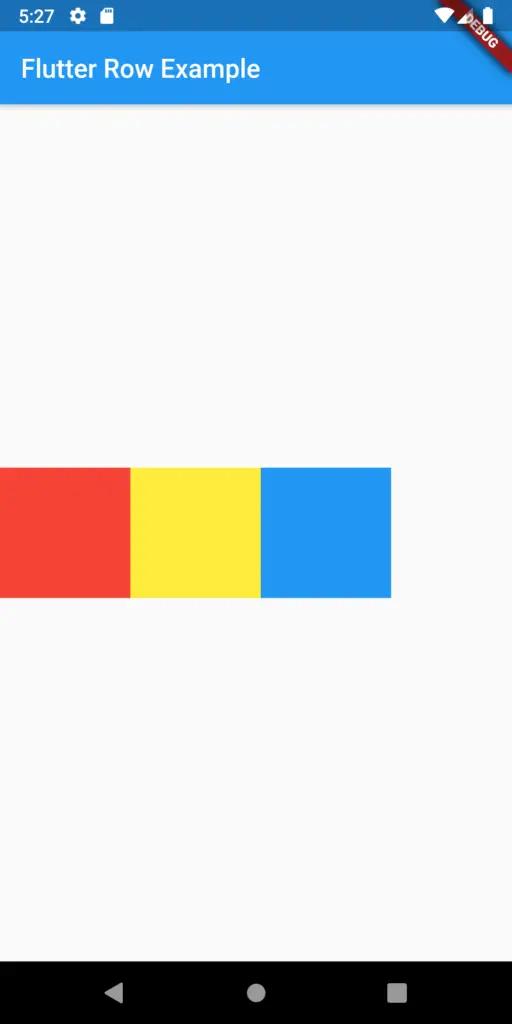
Similar to Row widget, Column widget is also easy to use.
Column(
children: <Widget>[
Container(
color: Colors.red,
height: 100,
width: 100,
),
Container(
color: Colors.yellow,
height: 100,
width: 100,
),
Container(
color: Colors.blue,
height: 100,
width: 100,
),
],
),
Following is the complete example of a Column widget in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Column Example',
),
),
body: Center(
child: Column(
children: <Widget>[
Container(
color: Colors.red,
height: 100,
width: 100,
),
Container(
color: Colors.yellow,
height: 100,
width: 100,
),
Container(
color: Colors.blue,
height: 100,
width: 100,
),
],
),
),
);
}
}
And here’s the output of the example.
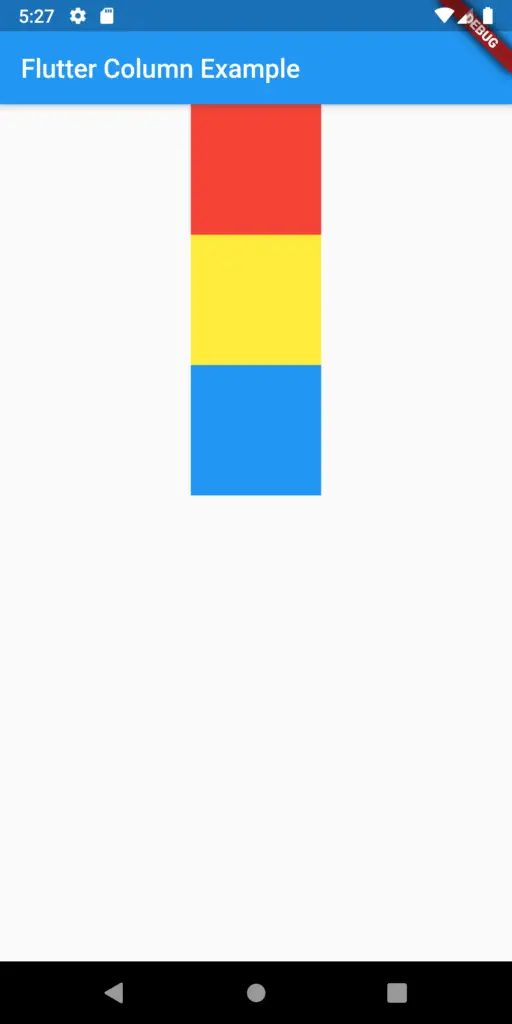
I hope this flutter tutorial helped you!