How to Align Widgets in Flutter
Proper alignment is an important aspect of a beautiful user interface. When the components are aligned properly, users can easily assess different options for your apps. In this blog post, let’s check how to align widgets in Flutter.
The Align widget and the Alignment property are used to align child widgets in Flutter. The Alignment property defines the coordinates to align the child. The following snippet aligns the Text widget centrally with respect to the parent.
const Align(
alignment: Alignment.center,
child: Text(
"Align Me!",
style: TextStyle(fontSize: 30.0),
),
)
Here’s the complete example:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter Center Alignment Example',
),
),
body: const Align(
alignment: Alignment.center,
child: Text(
"Align Me!",
style: TextStyle(fontSize: 30.0),
),
),
);
}
}
And the output will be as given below.
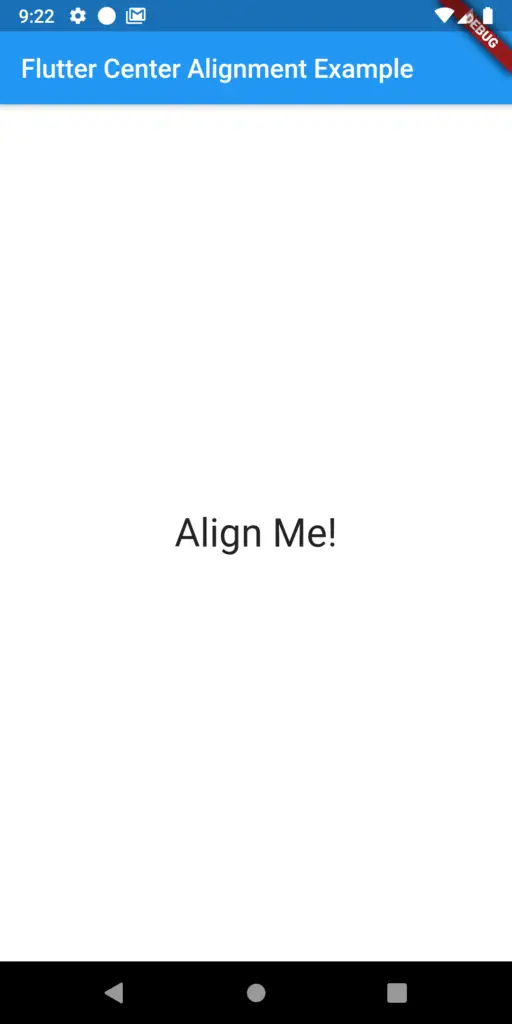
You can align to different positions by changing the value of the alignment property. If Alignment.center is replaced with Alignment.centerLeft you will get the following output.
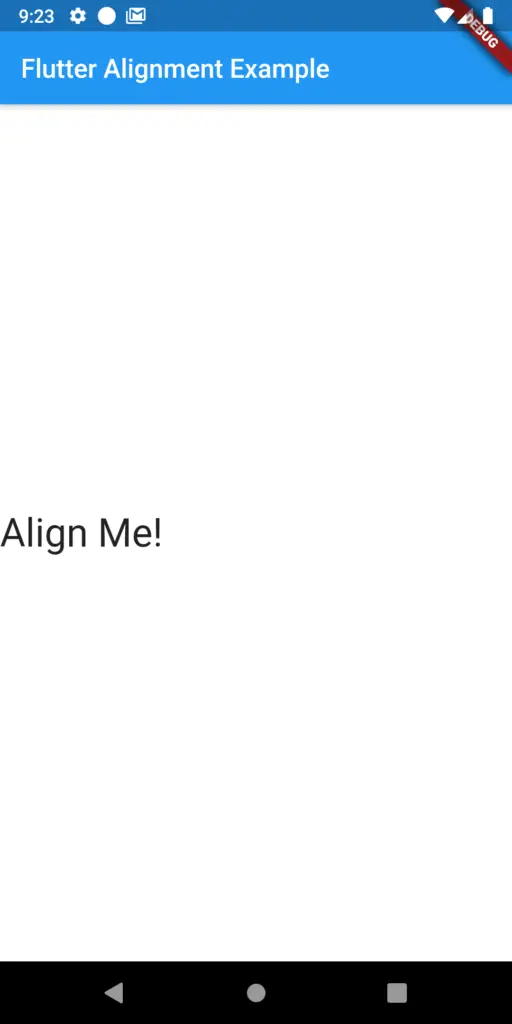
Alignment.centerRight
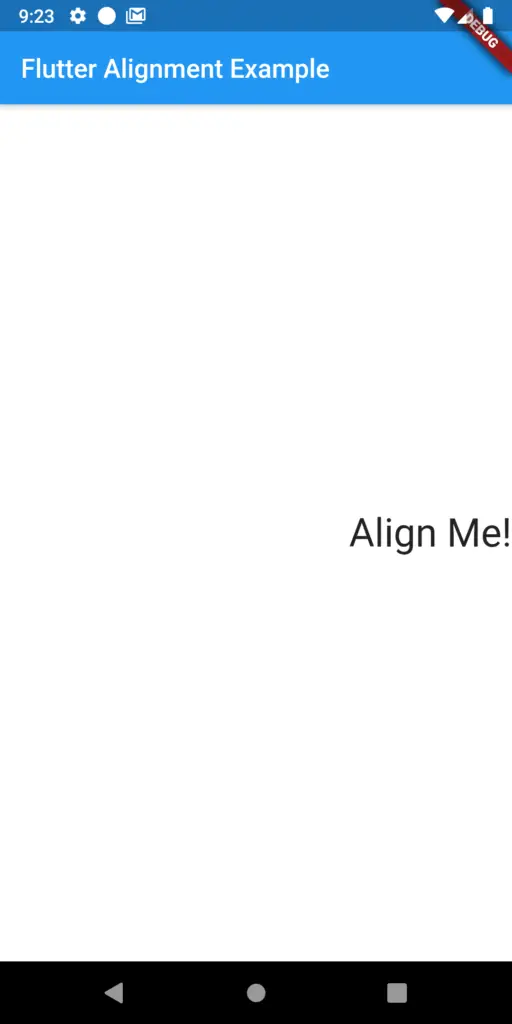
Alignment.topLeft
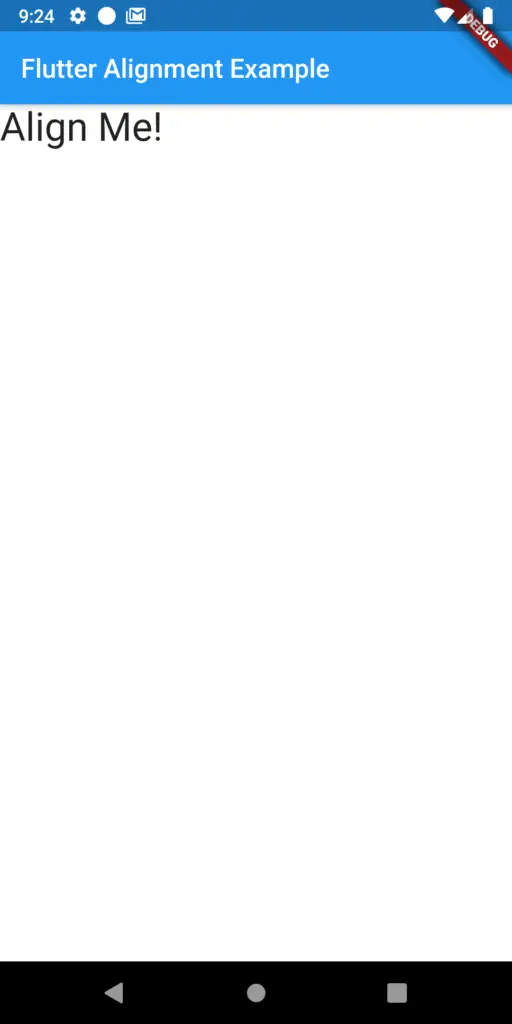
Alignment.topCenter
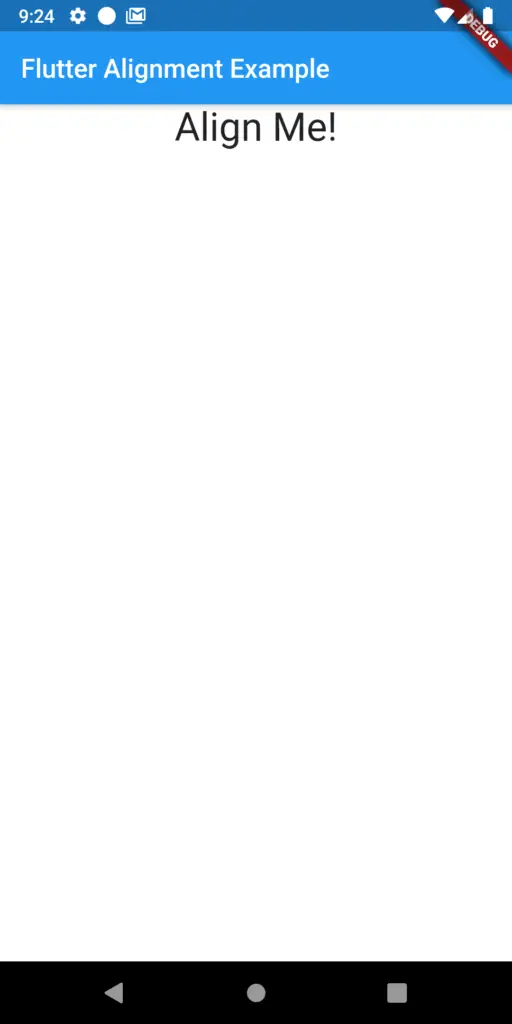
Alignment.topRight
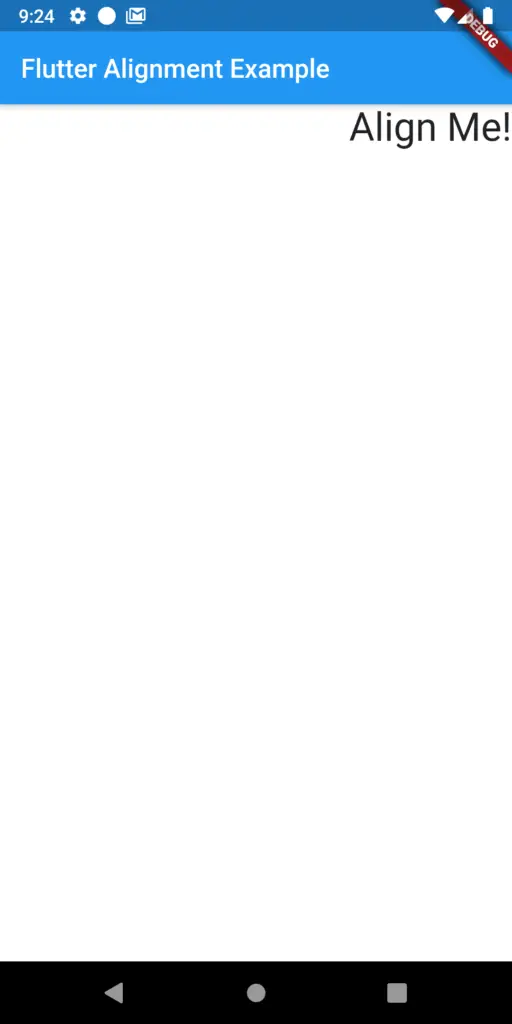
Alignment.bottomLeft
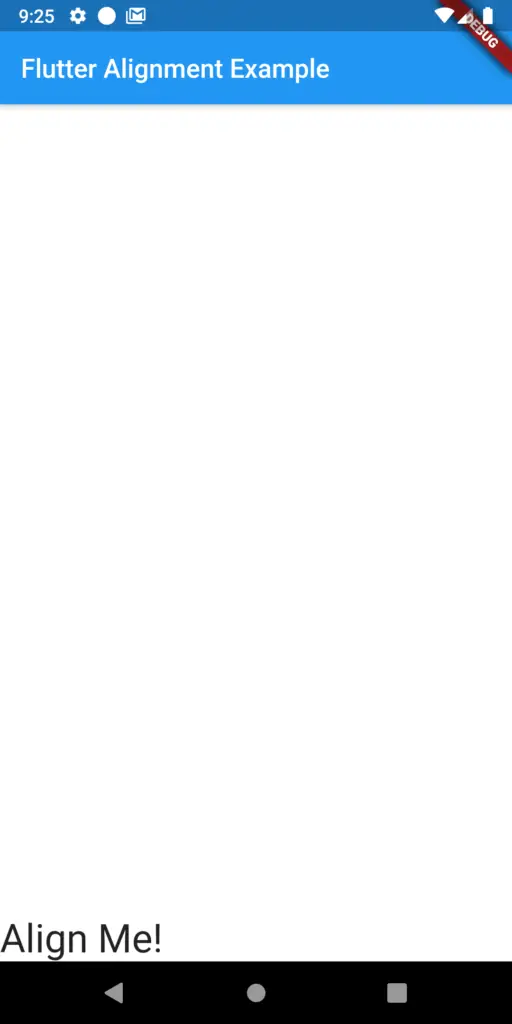
Alignment.bottomCenter
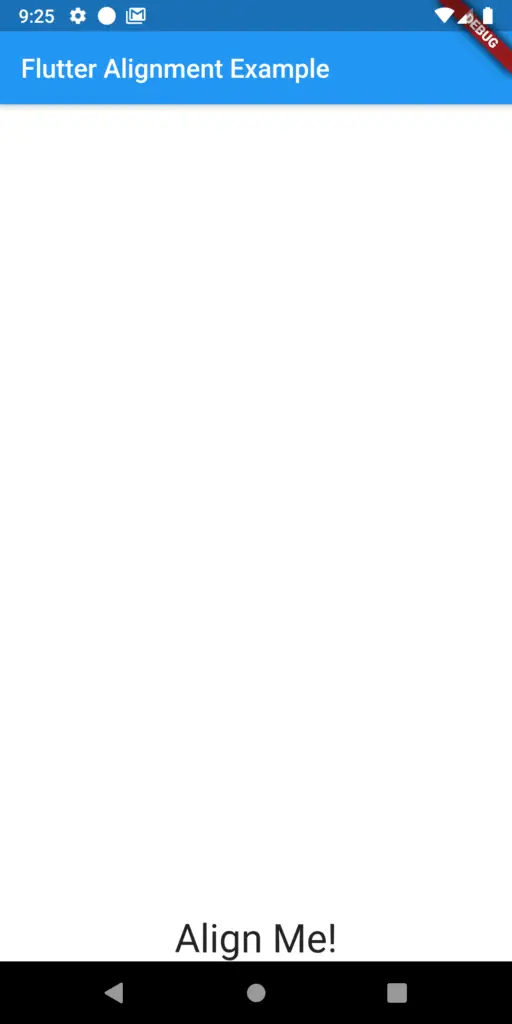
Alignment.bottomRight
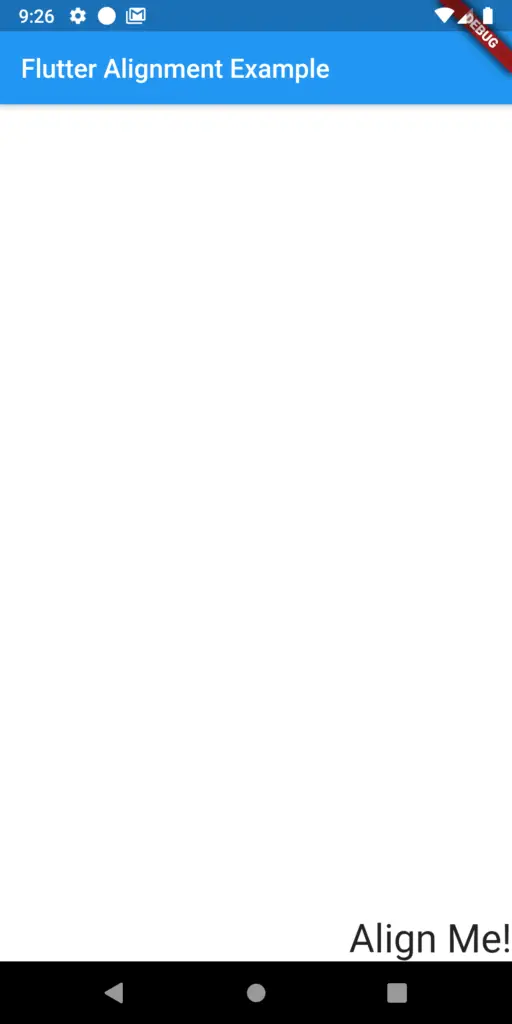
You can also align child widgets by defining custom coordinate points.
const Align(
alignment: Alignment(0.5, 0.5),
child: Text(
"Align Me!",
style: TextStyle(fontSize: 30.0),
),
)
And here’s the output:
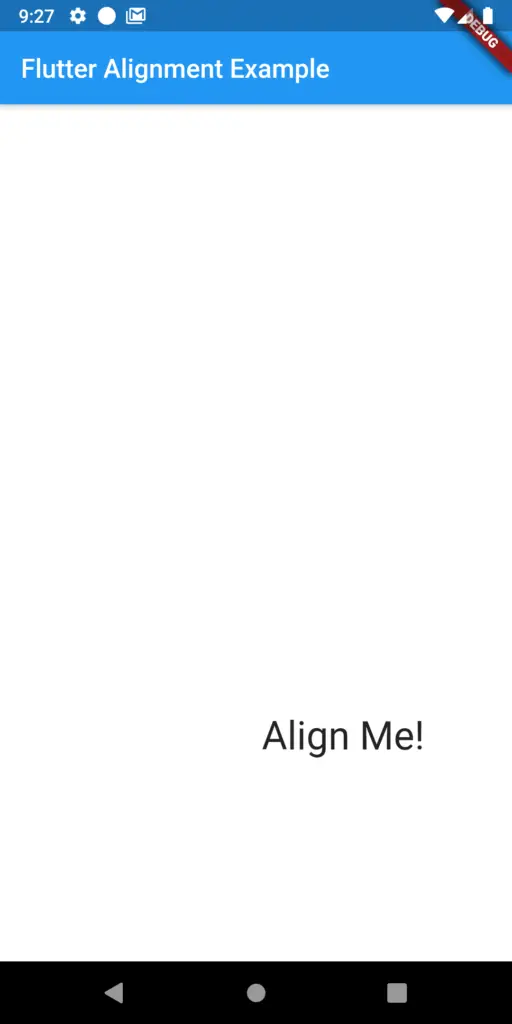
I hope this Flutter tutorial on alignment helped you!