How to Align TextField HintText to Center in Flutter
The importance of TextField in a mobile app doesn’t need any explanation. In this blog post, let’s learn how to align TextField to center in Flutter.
You can add hint text using InputDecoration class. In order to align hint text you have to make use of the Textfield property textAlign and the TextAlign class.
See the following code snippet.
TextField(
textAlign: TextAlign.center,
decoration: const InputDecoration(
hintText: 'Username',
border: OutlineInputBorder(),
),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
Note that aligning hint text to the center also results in aligning TextField text to the center.
The output is given below.
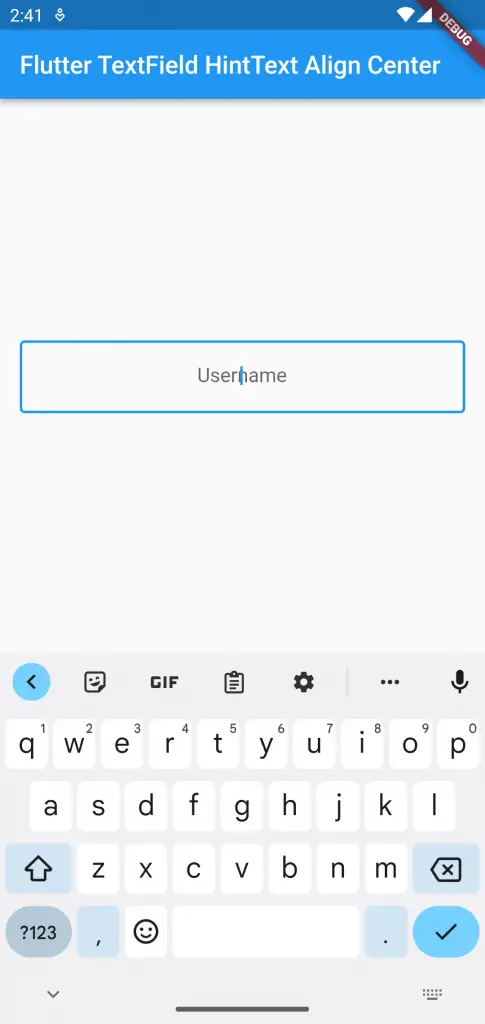
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField HintText Align Center'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
textAlign: TextAlign.center,
decoration: const InputDecoration(
hintText: 'Username',
border: OutlineInputBorder(),
),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
),
));
}
}
That’s how you align TextField hint text in Flutter.