How to Align AppBar Title to Center in Flutter
The AppBar widget appears at the top of each screen in a Flutter app. The title of AppBar is aligned to left by default in Android devices. Let’s check how to align AppBar text to the center in Flutter easily.
The AppBar class has a property title to add text. You can align this text to the center by making centerTitle property of the Appbar true. See the code snippet given below.
AppBar(
title: const Text(
'Flutter Appbar',
),
centerTitle: true,
),
You will get the output as given below.
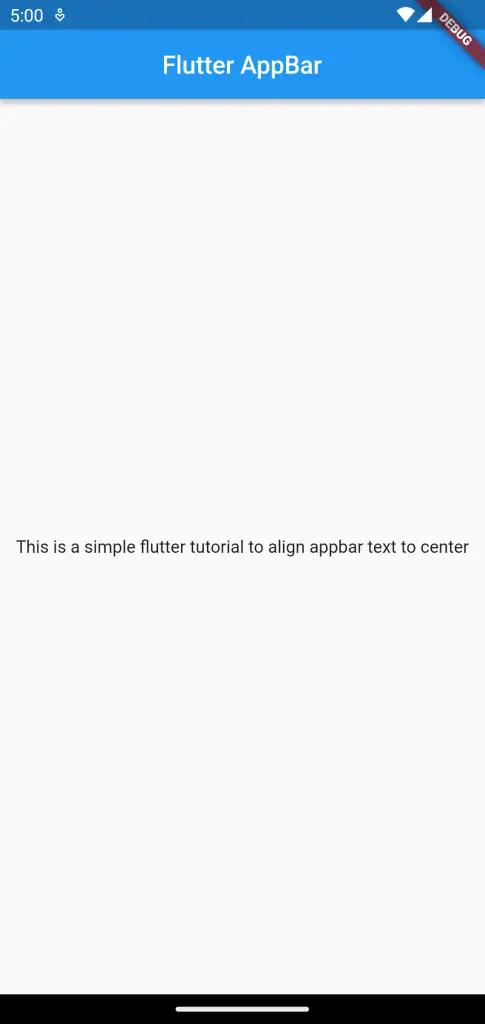
As you see, the title ‘Flutter AppBar’ is aligned to the center. Following is the complete code for reference.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Flutter AppBar',
),
centerTitle: true,
),
body: const Center(
child: Text(
'This is a simple flutter tutorial to align appbar text to center')));
}
}
That’s how you center the AppBar title in Flutter. I hope this short Flutter tutorial is helpful for you.