How to Add Time Picker in Flutter
Sometimes, we need specific time input from the users. Time pickers are used in such situations. In this blog post, let’s see how to add a time picker in Flutter.
I already have a blog post that shows how to add a date picker in flutter. Flutter has an inbuilt function named showTimePicker to display the time picker. It returns the selected date too.
showTimePicker(
initialTime: TimeOfDay.now(),
context: context);
Following is the complete Flutter time picker example. It displays a time picker when the user taps on the TextField widget. For that purpose, we use the onTap property of TextField. We make the readOnly property true so that the user can’t modify the text using the keyboard.
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
useMaterial3: true,
),
home: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
final timeController = TextEditingController();
@override
void dispose() {
// Clean up the controller when the widget is removed
timeController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Time Picker Example'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: TextField(
readOnly: true,
controller: timeController,
decoration: const InputDecoration(hintText: 'Pick your Time'),
onTap: () async {
var time = await showTimePicker(
context: context, initialTime: TimeOfDay.now());
if (time != null) {
timeController.text = time.format(context);
}
},
))));
}
}
Following is the output of the Flutter time picker example with TextField.
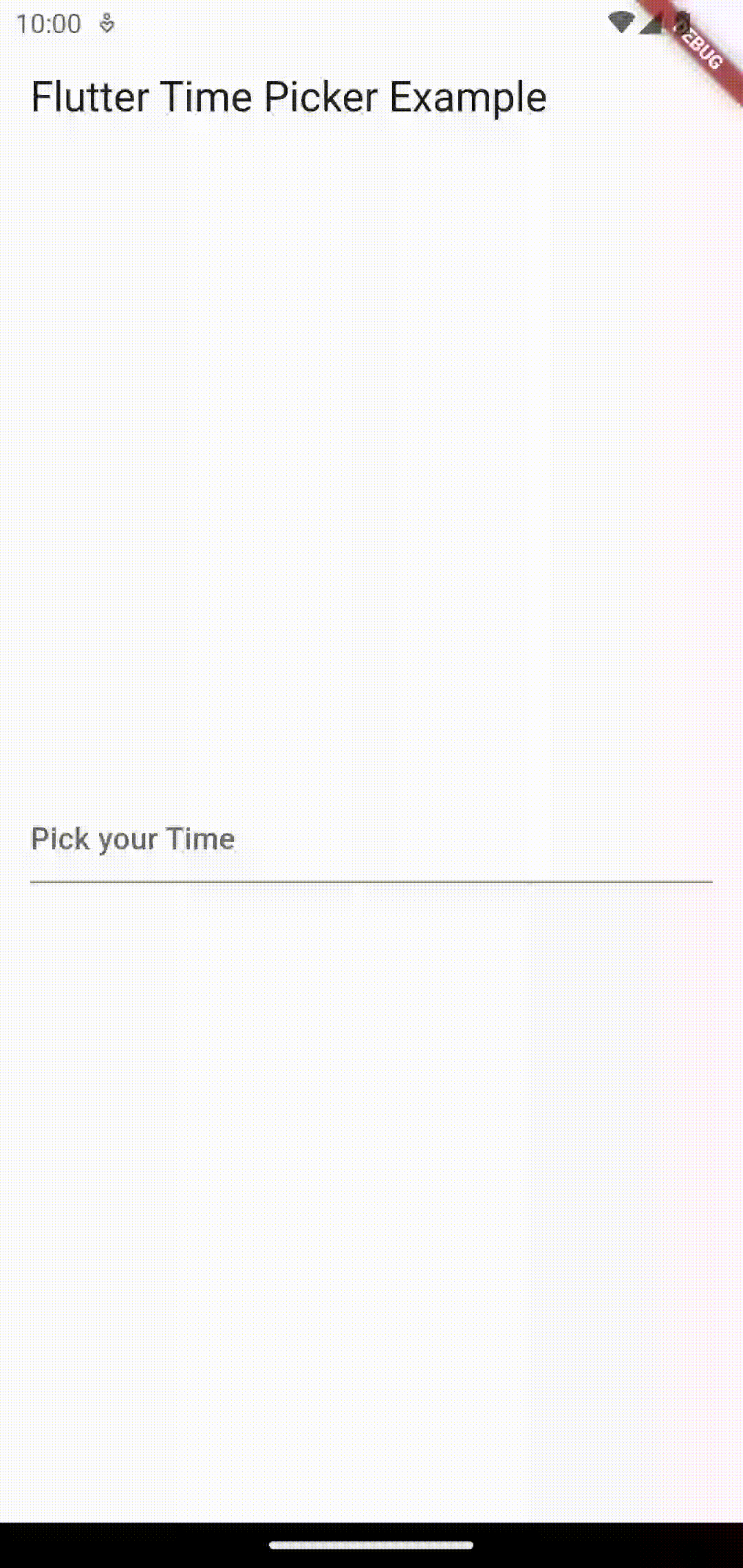
I hope this flutter tutorial will help you. Stay tuned for more flutter tutorials.