How to add TextField Floating Label in Flutter
Floating labels are beautiful labels that can be added to TextField. They help users to identify what to enter in the input. In this Flutter tutorial, let’s check how to add a floating label to the TextField widget.
Adding a floating label is easy and you just need to use the InputDecoration class. The labelText property is enough to add the floating label.
See the code snippet given below.
TextField(
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: 'Username',
),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
)
Then you will get the following output.
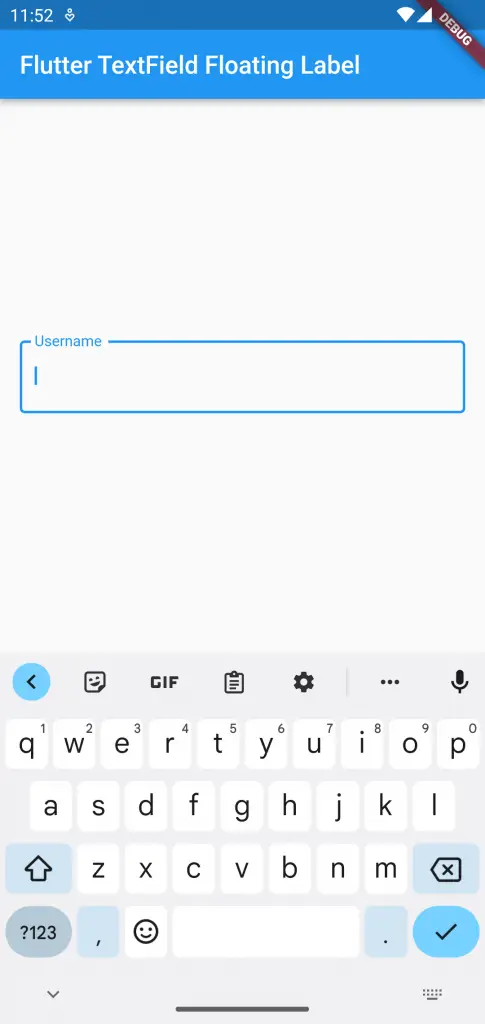
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Floating Label'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: TextField(
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: 'Username',
),
controller: _controller,
onSubmitted: (String value) {
debugPrint(value);
},
)));
}
}
That’s how to add a floating label to TextField in Flutter.