How to Add Placeholder Text in Flutter TextField
A placeholder text is a hint text which gives the user an idea about what to enter in the text input field. In this blog post, let’s see how to add placeholder text in Flutter TextField.
As I mentioned in my previous Flutter TextField tutorial, the TextField widget is used to create text inputs in Flutter. It has many useful properties. We can use the hintText property of the InputDecoration widget to show placeholder text.
See the code snippet given below.
TextField(
controller: _controller,
decoration:
const InputDecoration(hintText: 'Enter your name'),
onSubmitted: (String value) {
debugPrint(value);
},
),
Following is the complete example of the TextField with a placeholder text.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter TextField Placeholder'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
late TextEditingController _controller;
@override
void initState() {
super.initState();
_controller = TextEditingController();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: Center(
child: Column(children: <Widget>[
TextField(
controller: _controller,
decoration:
const InputDecoration(hintText: 'Enter your name'),
onSubmitted: (String value) {
debugPrint(value);
},
),
]),
)));
}
}
Following is the output of the Flutter TextField placeholder example.
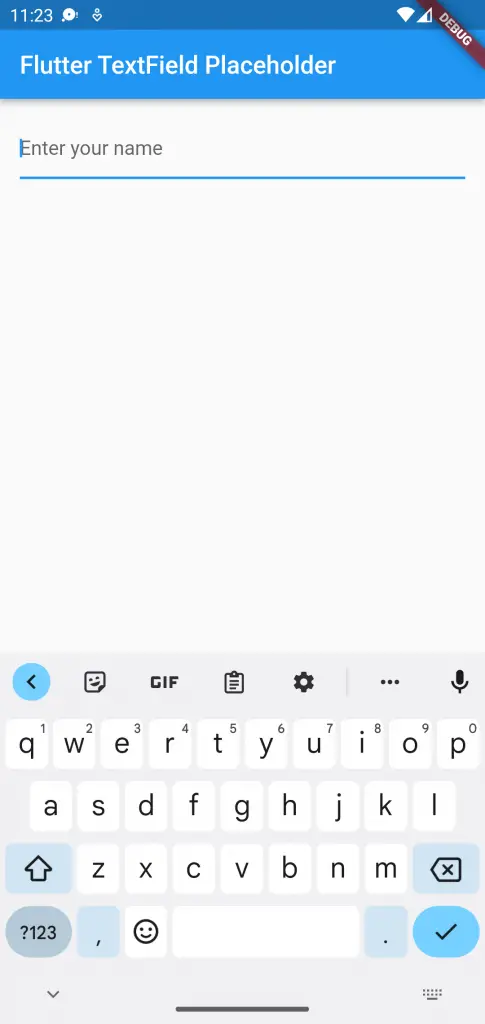
I hope this Flutter tutorial will help you. Thank you for reading!