How to add Margin to Card in Flutter
The Card is one of the popular material widgets used in Flutter. It comes with some default margin, which is the space around the card. In this tutorial, let’s learn how to change the Card margin in Flutter.
The default margin of the Card is 4.0 logical pixels. You can alter the margin using the margin property of the Card widget.
See the code snippet given below.
Card(
margin: const EdgeInsets.all(20),
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
The EdgeInsets class helps you to define the margins.
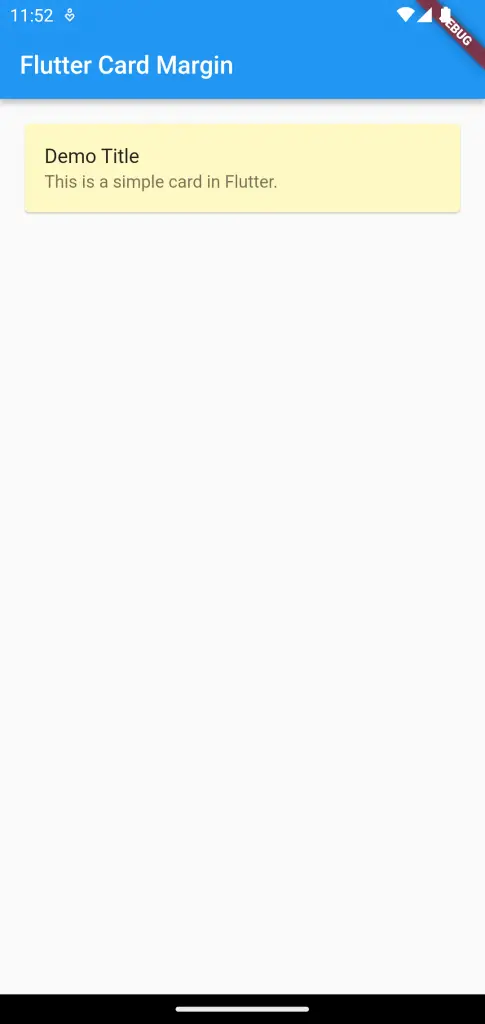
If you are using material 3 then you will get the following output.
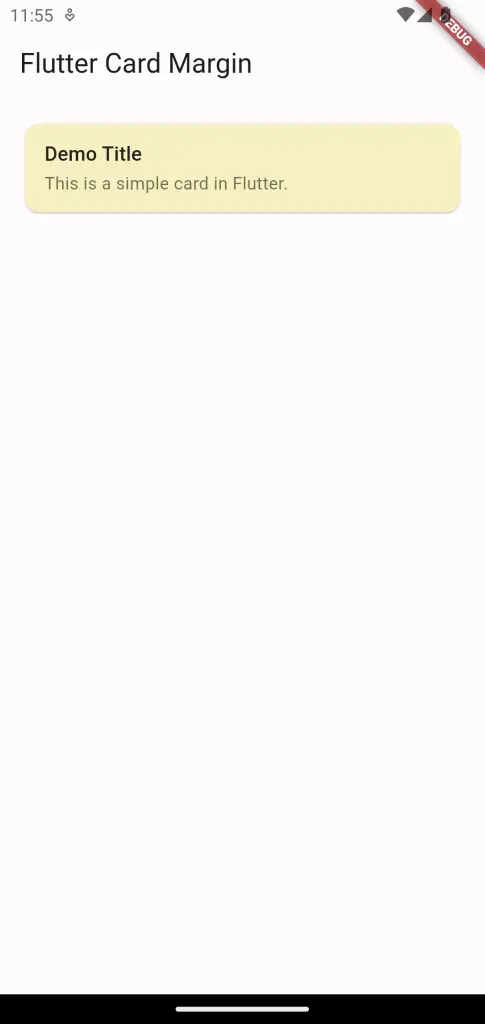
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
// colorSchemeSeed: const Color(0xff6750a4),
// useMaterial3: true,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Card Margin'),
),
body: Card(
margin: const EdgeInsets.all(20),
color: Colors.yellow[100],
child: Column(
mainAxisSize: MainAxisSize.min,
children: const <Widget>[
ListTile(
title: Text('Demo Title'),
subtitle: Text('This is a simple card in Flutter.'),
),
],
),
),
);
}
}
That’s how you change the Card margin in Flutter.