How to add Image with Rounded Corners in Flutter
Rounded corners are more beautiful than sharp corners. In this Flutter tutorial, let’s check how to add an image with rounded corners.
I already have a blog post on adding rounded corners in Flutter. As mentioned there, we have to use ClipRRect class to create rounded corners. It’s very easy. We just need to make our image the child of ClipRRect.
See the following code snippet.
ClipRRect(
borderRadius: BorderRadius.circular(20.0),
child: Image.network(
'https://cdn.pixabay.com/photo/2020/11/04/07/52/pumpkin-5711688_960_720.jpg'),
)
We are loading an image with rounded corners from the internet. The output is given below.
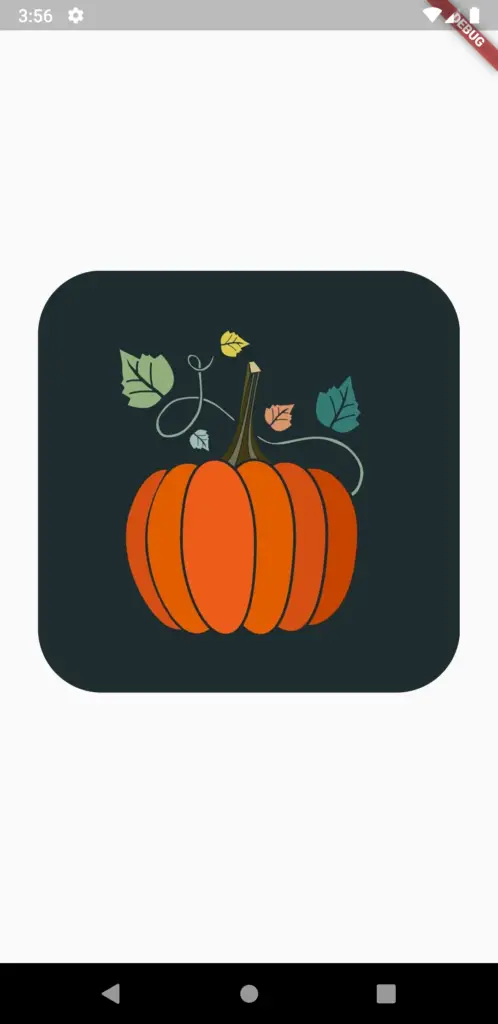
Following is the complete code for Flutter Image with rounded corners.
import 'package:flutter/material.dart';
void main() => runApp(App());
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Image with Rounded Corner Example',
home: FlutterExample(),
);
}
}
class FlutterExample extends StatelessWidget {
FlutterExample({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Padding(
padding: EdgeInsets.all(30),
child:ClipRRect(
borderRadius: BorderRadius.circular(50.0),
child: Image.network('https://cdn.pixabay.com/photo/2020/11/04/07/52/pumpkin-5711688_960_720.jpg'),
),),));
}
}
That’s how you add rounded corners to the Image in Flutter.