How to add Checkbox with Text and Icon in Flutter
The Checkbox is an important UI component in mobile apps. In this blog post, let’s learn how to create a Checkbox with text and icon in Flutter.
With the Checkbox widget and using other widgets, you can add text and icons. Here, we use the CheckboxListTile widget to create Checkbox with text and icon easily.
The CheckboxListTile widget is a mix of Checkbox widget and ListTile widget. The checkbox toggles when the user clicks anywhere on the tile.
You can add text using the title property whereas you can add icon using the secondary property. See the code snippet given below.
CheckboxListTile(
title: const Text('Checkbox with icon & text'),
secondary: const Icon(
Icons.face,
color: Colors.blue,
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
You will get the following output.
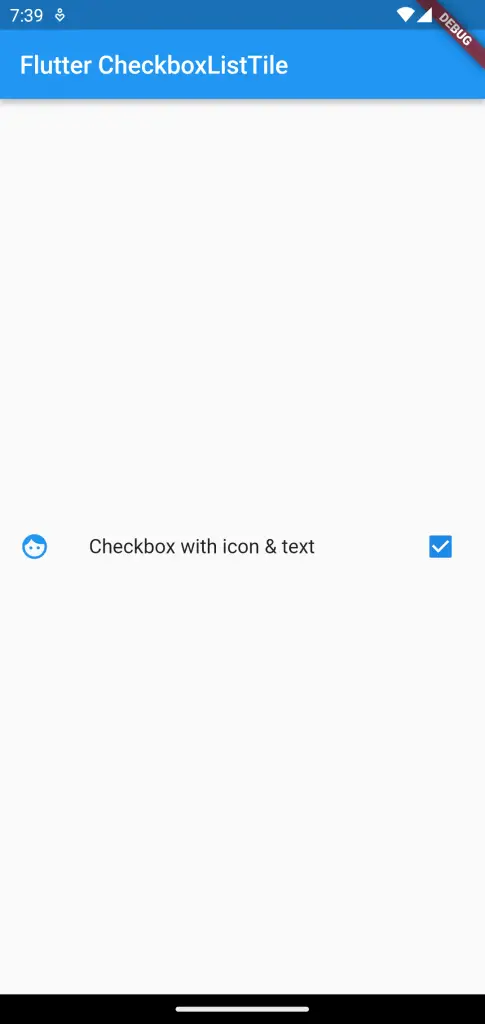
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter CheckboxListTile'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: CheckboxListTile(
title: const Text('Checkbox with icon & text'),
secondary: const Icon(
Icons.face,
color: Colors.blue,
),
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
);
}
}
That’s how you add checkbox with text and icon in Flutter easily.