How to add Border to Icon Button in Flutter
IconButton helps to add button functionalities to an icon in Flutter. Sometimes, we may want to add borders around icons. In this blog post, let’s learn how to add a border around IconButton in Flutter.
IconButton Circular Border
Here, I am using the Ink widget and adding IconButton as its child. The Ink widget helps to extend the pressable area of the IconButton to the borders. You should use its decoration property to draw a circular border with the help of CircleBorder class.
See the code snippet given below.
Ink(
decoration: const ShapeDecoration(
shape: CircleBorder(side: BorderSide(color: Colors.red, width: 2)),
),
child: IconButton(
icon: const Icon(Icons.favorite),
color: Colors.red,
iconSize: 50,
onPressed: () {},
),
)
This will give you a red circle border around the IconButton. The output is given below.
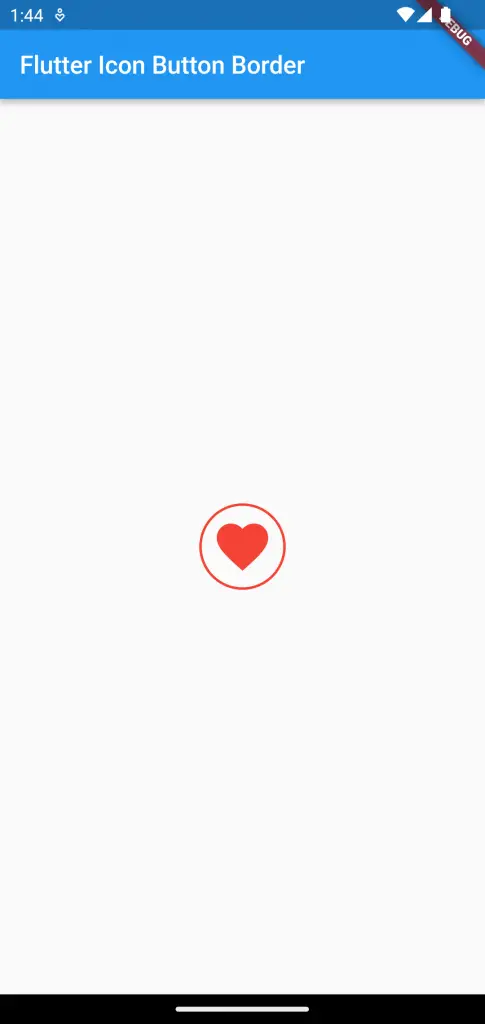
IconButton Rounded Rectangle Border
Sometimes, you may want rounded rectangle border instead of circular border. In such cases, you should use RoundedRectangleBorder class. See the code snippet given below.
Ink(
decoration: ShapeDecoration(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
side: const BorderSide(
color: Colors.red,
width: 2,
)),
),
child: IconButton(
icon: const Icon(Icons.favorite),
color: Colors.red,
iconSize: 50,
onPressed: () {},
),
)
You will get the following output with rounded rectangle border around the IconButton.
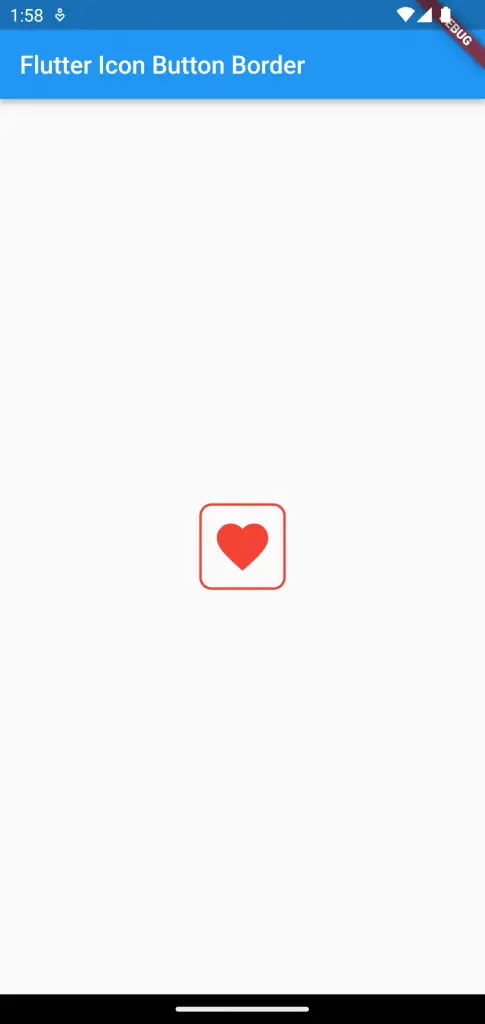
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Icon Button Border'),
),
body: Center(
child: Ink(
decoration: ShapeDecoration(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
side: const BorderSide(
color: Colors.red,
width: 2,
)),
),
child: IconButton(
icon: const Icon(Icons.favorite),
color: Colors.red,
iconSize: 50,
onPressed: () {},
),
)));
}
}
That’s how you add borders around IconButton in Flutter. I hope this tutorial is helpful for you.