How to add Border to CheckboxListTile in Flutter
The CheckboxListTile is a useful widget to add checkbox with text and icon. In this blog post, let’s learn how to add the CheckboxListTile border in Flutter.
The CheckboxListTile has many properties for customization. Using the shape property you can add a border, change the border color and even adjust the border radius.
See the code snippet given below.
CheckboxListTile(
title: const Text('Checkbox with text'),
shape: RoundedRectangleBorder(
side: const BorderSide(color: Colors.red, width: 5),
borderRadius: BorderRadius.circular(10)),
controlAffinity: ListTileControlAffinity.leading,
tileColor: Colors.yellow,
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
)
You will get the output of CheckboxListTile with red color border and yellow color background.
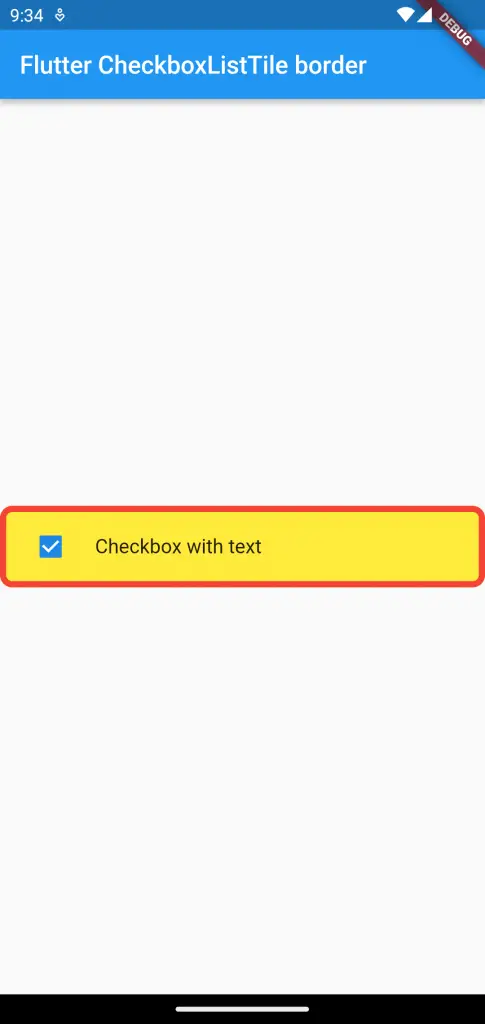
Following is the complete code.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter CheckboxListTile border'),
),
body: const MyStatefulWidget());
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({super.key});
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
bool checkBoxValue = false;
@override
Widget build(BuildContext context) {
return Center(
child: CheckboxListTile(
title: const Text('Checkbox with text'),
shape: RoundedRectangleBorder(
side: const BorderSide(color: Colors.red, width: 5),
borderRadius: BorderRadius.circular(10)),
controlAffinity: ListTileControlAffinity.leading,
tileColor: Colors.yellow,
value: checkBoxValue,
onChanged: (bool? newValue) {
setState(() {
checkBoxValue = newValue!;
});
},
),
);
}
}
That’s how you add the CheckboxListTile border in Flutter.